- Published on Friday, July 28, 2023, last updated
JS code coverage tool in 2023 - Istanbul vs Jest vs JS Coverage vs CodeCov
Table of Contents
- JS Code coverage
- 1.1 What is Code Coverage?
- 1.2 Code coverage limitations
- 1.3 Importance of Code Coverage in JavaScript
- 2. Code Coverage Tools for JavaScript: An Overview
- 2.1 Criteria for Selecting a Code Coverage Tool
- 3. Detailed Review of Selected Tools
- 3.1 Tool 1: Istanbul
- 3.2 Tool 2: Jest
- 3.3 Tool 3: JS Coverage
- 3.4 Tool 4: CodeCov
- 4. Comparison of the Tools
- 4.1 Comparison Table: Istanbul vs Jest vs JS Coverage vs CodeCov
- 4.2 Which Tool to Choose and When?
JS Code coverage
Maintaining the quality and reliability of code is crucial. One of the key metrics that helps in this process is 'Code Coverage'. This article will focus on the concept of Code Coverage, its importance in JavaScript, and explore some of the best tools available for measuring it.
1.1 What is Code Coverage?
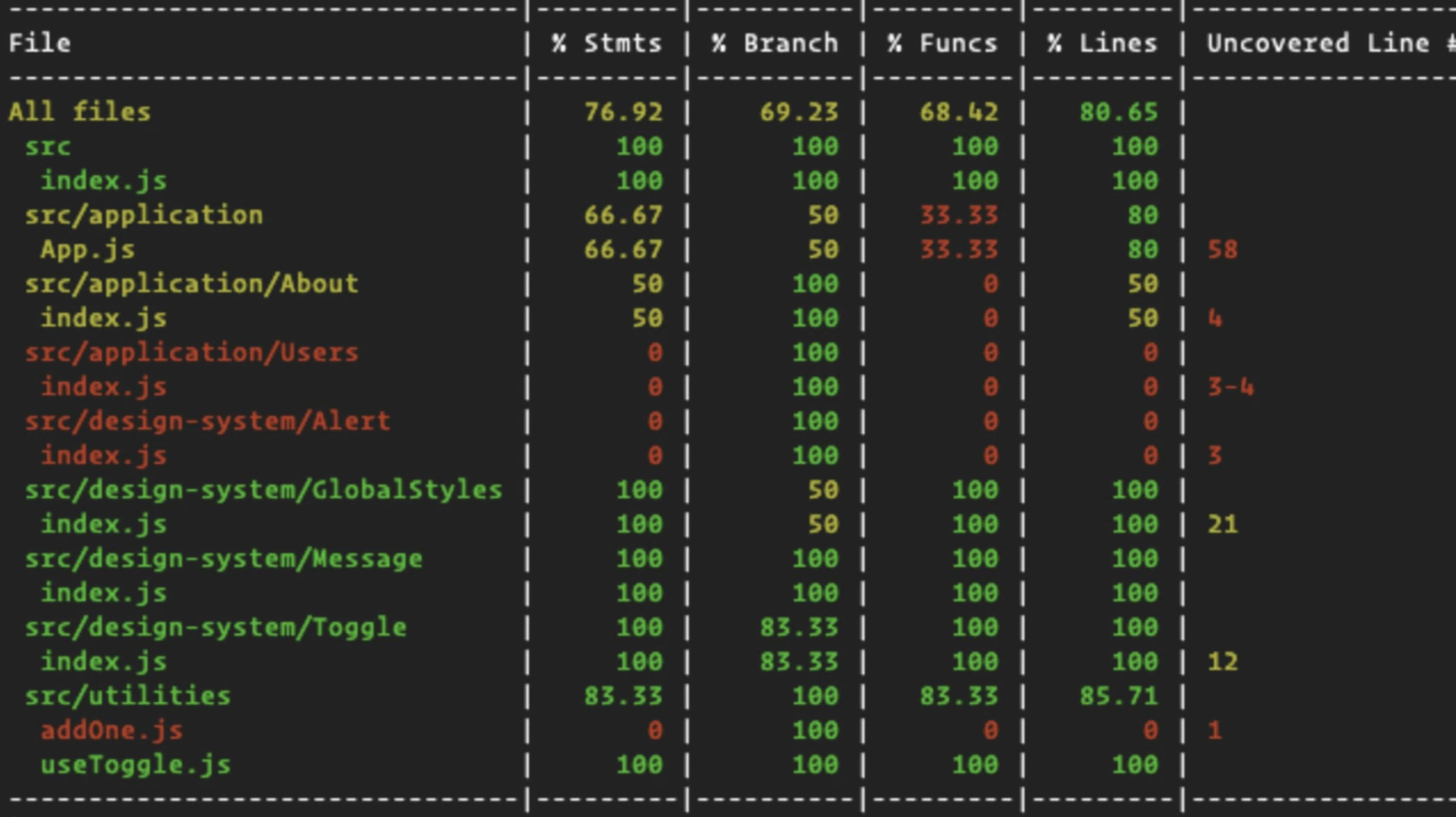
Code Coverage is a metric that quantifies the degree to which the source code of a program is executed during testing. It is typically measured as a percentage, with higher percentages indicating that more of the source code has been covered by the tests. This metric can help identify areas of the code that have not been tested and need further attention.
1.2 Code coverage limitations
While code coverage is a valuable tool in software testing, it's important to understand its limitations. Code coverage measures the extent of code that is executed during testing, but it doesn't assess the quality of the tests themselves. High code coverage can give a false sense of security if the tests are poorly written or if they don't adequately check for correct outcomes. Furthermore, code coverage doesn't account for different paths through the code that might occur under varying conditions. It also can't guarantee that all possible inputs or states of your application are tested. Therefore, while striving for high code coverage is beneficial, it should be complemented with other testing strategies and quality assurance practices to ensure comprehensive and effective testing.
“High code coverage can give a false sense of security if the tests are poorly written or if they don't adequately check for correct outcomes. ”
— ...doesn't account for different paths through the code that might occur under varying conditions....it should be complemented with other testing strategies and quality assurance practices to ensure comprehensive and effective testing.
1.3 Importance of Code Coverage in JavaScript
JavaScript, as one of the most widely used programming languages, forms the backbone of many web applications. Ensuring the reliability of JavaScript code is therefore vital for the overall performance and user experience of these applications.
In the context of JavaScript, Code Coverage serves as a visual measurement that helps developers identify untested parts of the code. This can prevent potential bugs from making their way into production and aids in maintaining and improving the quality of the code over time.
In the following sections, we will delve into some of the top tools for measuring Code Coverage in JavaScript, providing a comprehensive analysis of their features, pros, cons, and usage.
Axolo User Experiences
2480+ developers online
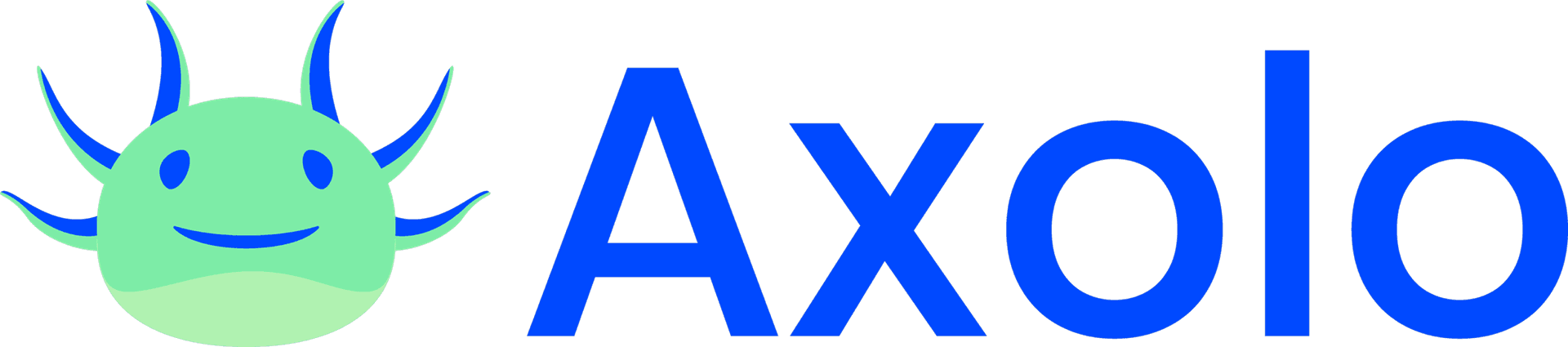
2. Code Coverage Tools for JavaScript: An Overview
In the JavaScript ecosystem, there are several tools available that can help developers measure code coverage. These tools vary in their features, ease of use, and the level of detail they provide about the code. Some tools are standalone code coverage tools, while others are testing frameworks that include code coverage functionality. In this section, we will provide an overview of these tools and discuss the criteria for selecting a code coverage tool.
2.1 Criteria for Selecting a Code Coverage Tool
Choosing the right code coverage tool can significantly impact the effectiveness of your testing process. Here are some key criteria to consider when selecting a code coverage tool for JavaScript:
Compatibility: The tool should be compatible with your tech stack. It should work seamlessly with your existing testing frameworks and libraries.
Ease of Use: The tool should be user-friendly and easy to integrate into your existing workflow. It should provide clear and understandable reports.
Detailed Reporting: Look for tools that offer detailed and comprehensive reports. The reports should not only show the percentage of code covered but also indicate which parts of the code are not covered.
Performance: The tool should not significantly slow down your testing process. It should be efficient and fast.
Community Support: Tools with strong community support tend to have regular updates, more resources for learning, and quicker bug fixes.
Integration with CI/CD: If you use continuous integration/continuous deployment (CI/CD), the tool should ideally integrate with your CI/CD system to automate the code coverage process.
By considering these criteria, you can select a code coverage tool that best fits your project's needs and contributes to the overall quality of your code.
3. Detailed Review of Selected Tools
3.1 Tool 1: Istanbul
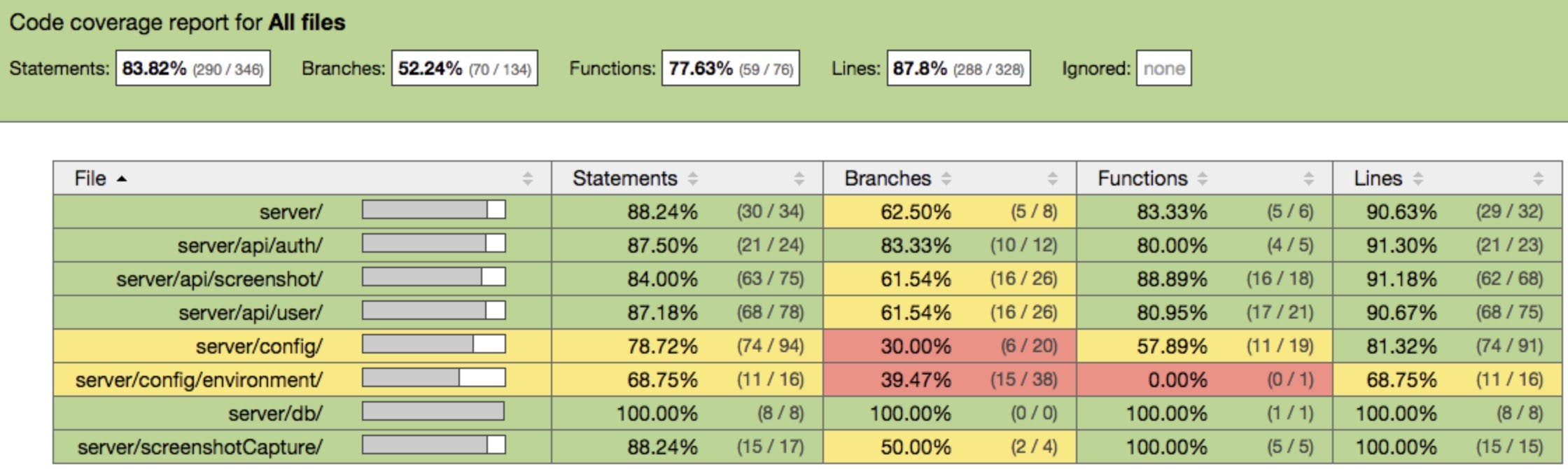
3.1.1 Overview of Istanbul
Istanbul, also known as nyc, is a state-of-the-art command-line interface for JavaScript code coverage. It instruments your ES5 and ES2015+ JavaScript code with line counters, allowing you to track how well your unit-tests exercise your codebase. The nyc command-line client for Istanbul works well with most JavaScript testing frameworks like tap, mocha, AVA, etc.
3.1.2 Features of Istanbul
Istanbul supports applications that spawn subprocesses. It provides source-mapped coverage of Babel and TypeScript projects. Istanbul allows you to set custom coverage thresholds that will fail if check-coverage is set to true and your coverage drops below those thresholds. It supports both inline source-maps and .map files for pre-instrumented codebases. Istanbul can combine the coverage report for you if configured correctly with the --no-clean flag and the report command.
3.1.3 Pros and Cons of Istanbul
👍 Pros:
Istanbul supports a wide variety of configuration arguments. It allows you to inherit other configurations using the key extends in the package.json stanza, .nycrc, or YAML files. Istanbul supports caching of instrumented files to disk to prevent instrumenting source files multiple times, and speed nyc execution times.
👎 Cons:
One potential downside could be the complexity of configuration for beginners.
3.1.4 How to Use Istanbul for Code Coverage
To use Istanbul for code coverage, you need to add it as a dev dependency using your package manager: npm i -D nyc or yarn add -D nyc. You can use nyc to call npm scripts (assuming they don't already have nyc executed in them).
For example:
{
"scripts": {
"test": "mocha",
"coverage": "nyc npm run test"
}
}
You can also use npx instead of installing nyc as a dependency, but you might get updates you are not ready for; to get around this, pin to a specific major version by specifying, e.g. nyc@14.
For more detailed instructions and advanced usage, you can refer to the Istanbul .
3.2 Tool 2: Jest
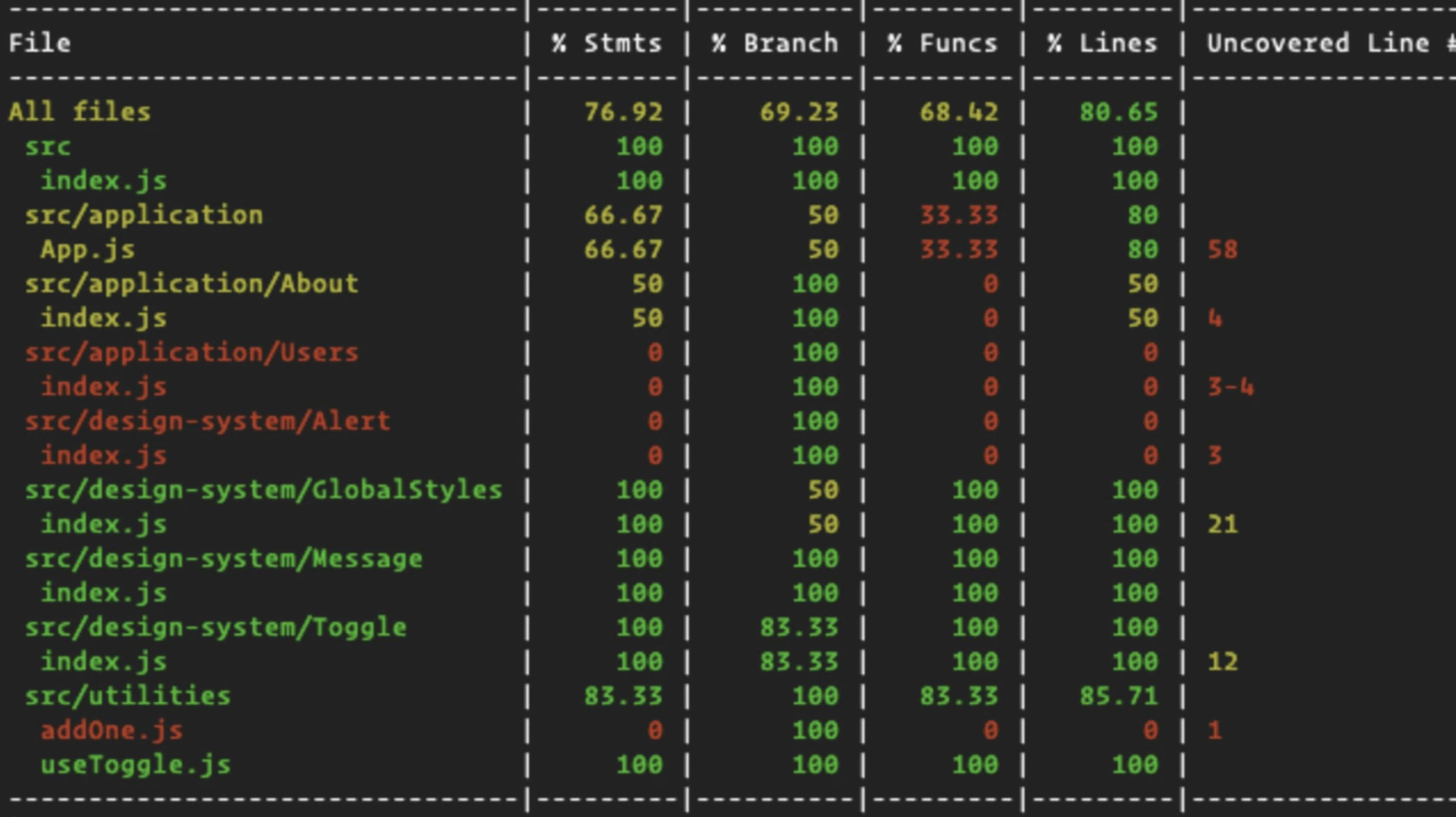
3.2.1 Overview of Jest
Jest is a delightful JavaScript testing framework developed and regularly maintained by Facebook. It works out of the box for most JavaScript projects and provides an instant feedback loop to developers. Jest is known for its "zero-configuration" philosophy. You don't have to make a lot of decisions and tweak configurations to get started with Jest. It also supports snapshot testing, which can capture snapshots of large objects to simplify testing and analyze how they change over time.
3.2.2 Features of Jest
Zero-configuration: Jest works out of the box for most JavaScript projects, which means you can get started quickly without having to decide on a lot of configurations. Snapshot Testing: Jest can capture snapshots of React trees or other serializable values to write tests quickly and provide a way to track changes over time. Interactive Watch Mode: Jest's watch mode runs only the tests related to the files changed since the last commit, providing a fast feedback loop. Mocking and Isolation: Jest allows you to mock objects in your test files. It supports function mocking, manual mocking, timer mocking, and more. Code Coverage: With Jest, you can collect code coverage information and see exactly which lines of your code are being tested.
3.2.3 Pros and Cons of Jest
👍 Pros:
Jest is easy to set up and use, making it a good choice for projects of any size. It has a rich API for various features like mocking and spying. Jest has strong community support and is regularly maintained by Facebook.
👎 Cons:
Jest runs slower compared to some other testing libraries like Mocha. While Jest's snapshot testing can be useful, it can also lead to large snapshot files that need to be manually reviewed and maintained.
3.2.4 How to Use Jest for Code Coverage
To use Jest for code coverage, you need to install Jest as a dev dependency in your project:
npm install --save-dev jest
Or if you are using yarn:
yarn add --dev jest
Then, you can add a script in your package.json to run your tests with Jest:
{
"scripts": {
"test": "jest"
}
}
To collect code coverage, you can run Jest with the --coverage flag:
npm test -- --coverage
Or with yarn:
yarn test --coverage
This will generate a coverage report in a new directory, coverage, in your project root.
For more detailed instructions and advanced usage, you can refer to the .
3.3 Tool 3: JS Coverage
3.3.1 Overview of JS Coverage
JS Coverage is a code coverage tool written in pure JavaScript, supporting both Node.js and JavaScript. It is designed to be easy to use and flexible, allowing developers to quickly and efficiently measure the code coverage of their JavaScript code.
3.3.2 Features of JS Coverage
- JS Coverage supports both Node.js and JavaScript.
- It provides a command-line interface for easy use and integration into testing workflows.
- JS Coverage supports the injection of functions into your module exports for easy testing.
- It allows for the creation of coverage reports in various formats, including spec, list, tap, detail, and HTML.
- JS Coverage supports the use of a
.covignore
file to specify files to be ignored during coverage calculation.
3.3.3 Pros and Cons of JS Coverage
👍 Pros:
- JS Coverage is easy to use and flexible, making it a good choice for projects of any size.
- It supports a wide variety of report formats, allowing developers to choose the one that best suits their needs.
- JS Coverage supports the use of a
.covignore
file, providing flexibility in determining which files should be included in the coverage calculation.
👎 Cons:
- The documentation for JS Coverage is somewhat limited, which may make it harder for new users to get started.
- JS Coverage has not been updated recently (as of July 2023), which may indicate a lack of ongoing maintenance and support.
3.3.4 How to Use JS Coverage for Code Coverage
To use JS Coverage for code coverage, you need to install it as a dependency in your project:
npm install jscoverage
Then, you can use JS Coverage to instrument your JavaScript files for coverage measurement:
jscoverage source.js source-cov.js
This will create a new file, source-cov.js, which is an instrumented version of source.js that can be used to measure code coverage.
For more detailed instructions and advanced usage, you can refer to the .
3.4 Tool 4: CodeCov
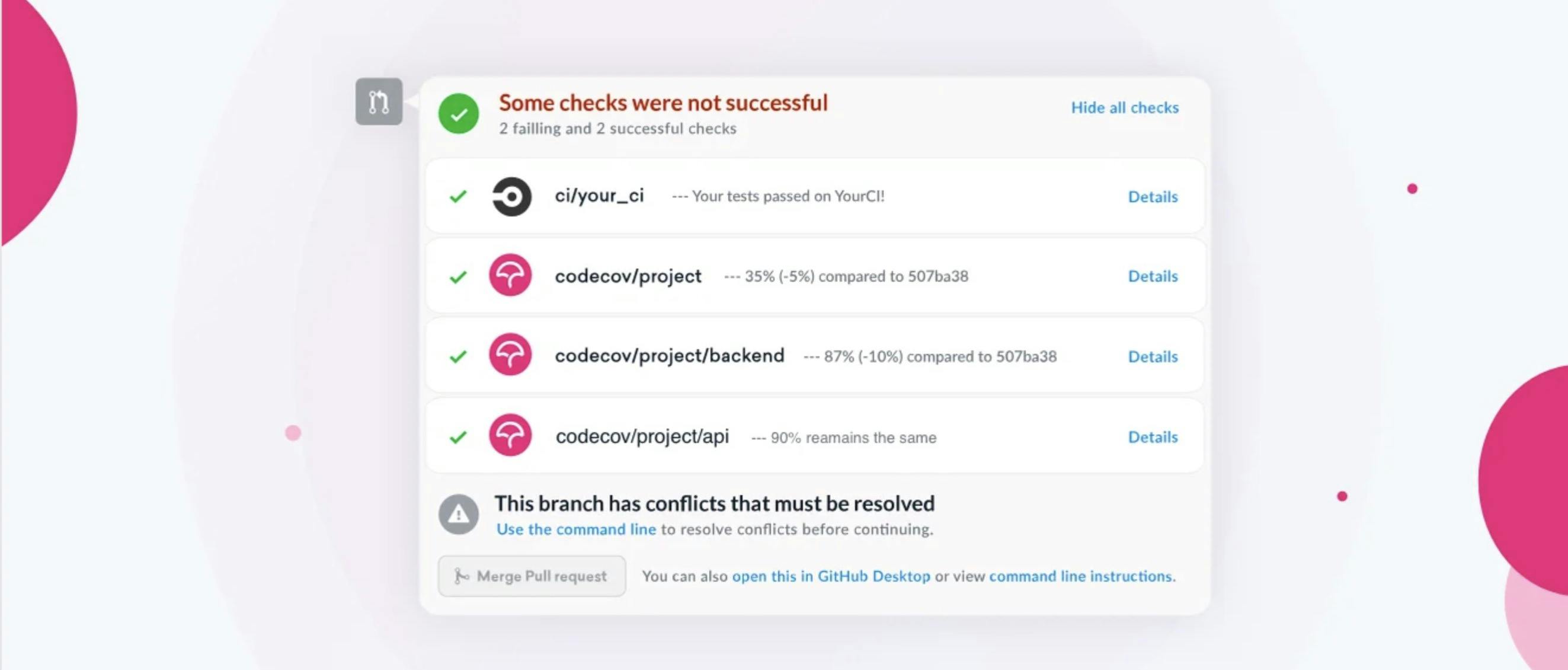
3.4.1 Overview of CodeCov
CodeCov is a highly integrated tool that provides code coverage metrics to help developers write better code. It can be used with any testing framework, CI/CD service, and supports over 30 languages. CodeCov makes it easy to implement code coverage to your development workflow, providing team and repository reporting, pull request comments, and status checks.
3.4.2 Features of CodeCov
- CodeCov supports over 30 languages and can be used with any CI/CD service.
- It provides features like team management, repository reporting, pull request comments, and status checks.
- CodeCov offers a browser extension that overlays coverage reports on GitHub.
- It provides a variety of graphs and reports to visualize coverage data.
3.4.3 Pros and Cons of CodeCov
👍 Pros:
- CodeCov is highly integrated and flexible, making it a good choice for teams using CI/CD services.
- It provides detailed reports and visualizations, helping you understand your coverage data at a glance.
- CodeCov supports a wide range of languages, making it a versatile tool for multi-language projects.
👎 Cons:
- CodeCov is a paid tool, although it offers a free tier for open-source projects. The cost could be a barrier for some teams.
- The setup process can be complex compared to some other tools.
3.4.4 How to Use CodeCov for Code Coverage
To use CodeCov for code coverage, you need to sign up for a CodeCov account and add your repository. Once your repository is connected, you can add a CodeCov step to your CI/CD pipeline to upload your coverage reports. CodeCov will process the reports and provide you with detailed coverage metrics.
For more detailed instructions and advanced usage, you can refer to the .
4. Comparison of the Tools
4.1 Comparison Table: Istanbul vs Jest vs JS Coverage vs CodeCov
Feature | Istanbul | Jest | JS Coverage | CodeCov |
---|---|---|---|---|
Ease of Use | 🟢 | 🟢 | 🟡 | 🟢 |
Detailed Reporting | ✔️ | ✔️ | ✔️ | ✔️ |
Performance | 🟢 | 🟡 | 🟢 | 🟢 |
Community Support | 🟢 | 🟢 | ❌ | 🟢 |
CI/CD Integration | ✔️ | ✔️ | ❌ | ✔️ |
Latest Update (as of July 2023) | Recent | Recent | Not recent | Recent |
Pricing | Free | Free | Free | Free/Open Source, $10/user/month (Pro), Custom (Enterprise) |
4.2 Which Tool to Choose and When?
The choice of a code coverage tool depends largely on your specific needs and the nature of your project. Here are some general recommendations:
Istanbul: Choose Istanbul if you want a high-performance tool with detailed reporting and strong community support. It's a good choice for larger projects or projects where performance is a key concern.
Jest: Jest is a great choice if you're looking for a comprehensive testing solution that includes code coverage. It's easy to use and has strong community support, but it might be slower than some other tools.
JS Coverage: JS Coverage could be the right tool for you if you're working with a smaller project or if you prefer a tool that's easy to use and flexible. However, keep in mind that it hasn't been updated recently and doesn't have as much community support as the other tools.
CodeCov: CodeCov is a great option if you're looking for a tool that integrates well with CI/CD systems. It's easy to use, provides detailed reporting, and has strong community support.