- Published
Open Current GitHub Pull Requests from Your Terminal: Step-by-Step Guide for iTerm and Zsh
- Authors
- Name
- Sydney Cohen
- @chnsydney
Let's create a custom script for your zsh terminal to automatically open the current pull request in GitHub for the repository you're working in. If there are no corresponding pull request it will ask you to create a pull request on GitHub.
Here is a gif on how it will work eventually:
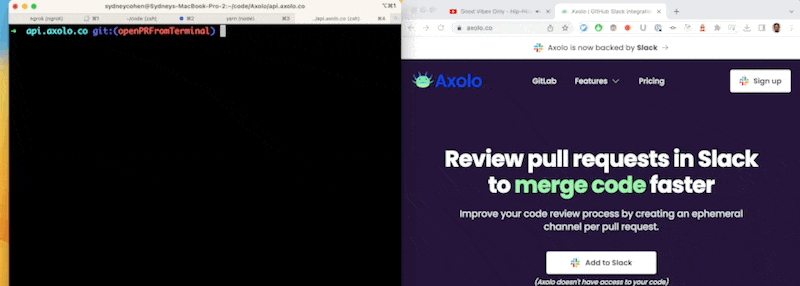
Here's a step-by-step guide:
1. Make sure you have jq and hub installed, as they will be required for parsing JSON and interacting with GitHub:
# Install jq
brew install jq
2. Install hub
brew install hub
3. Create a new file named open-pr.sh in your preferred scripts directory, for example, ~/.scripts/open-pr.sh.
4. Add the following script to the open-pr.sh file:
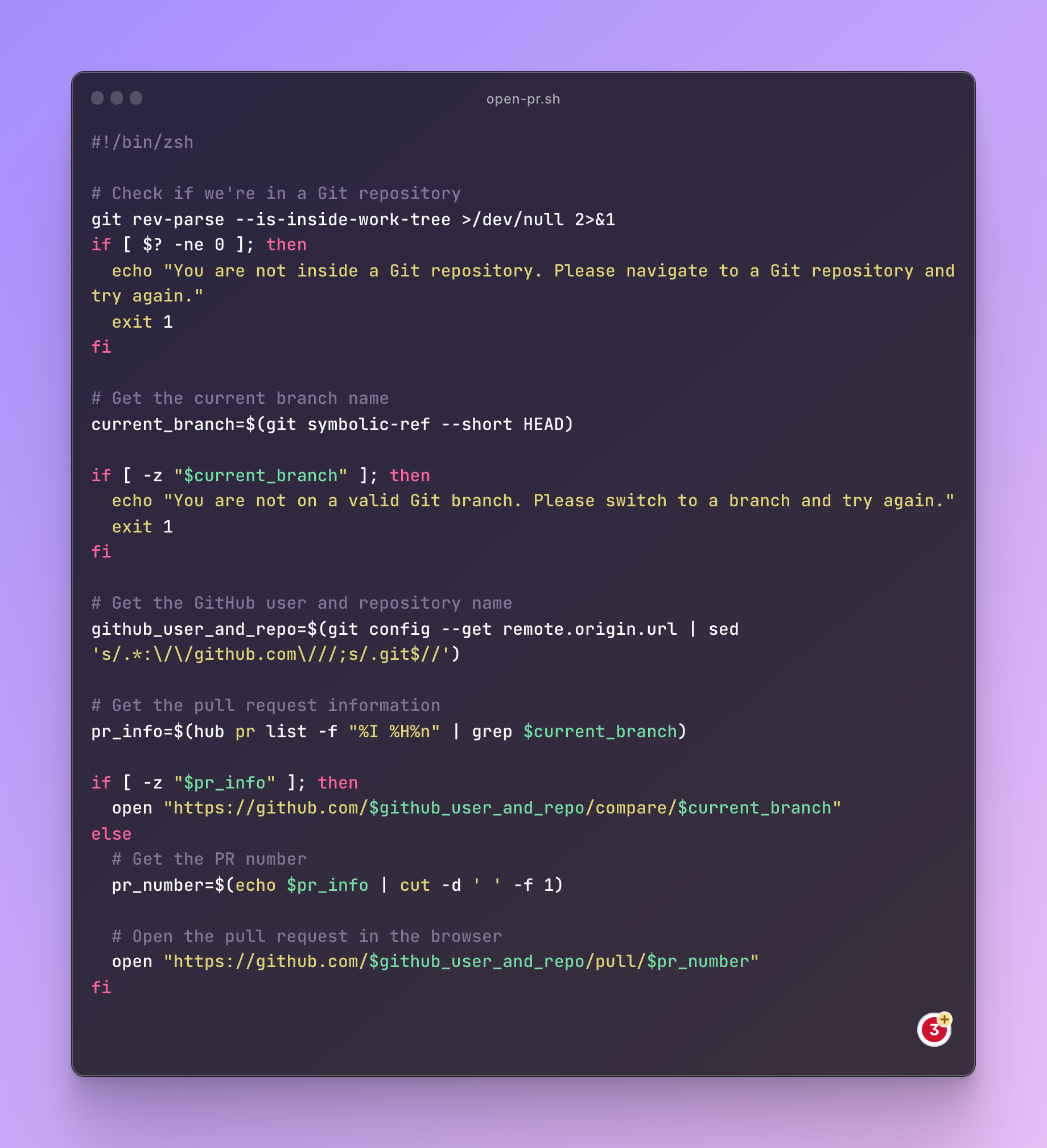
#!/bin/zsh
# Check if we're in a Git repository
git rev-parse --is-inside-work-tree >/dev/null 2>&1
if [ $? -ne 0 ]; then
echo "You are not inside a Git repository. Please navigate to a Git repository and try again."
exit 1
fi
# Get the current branch name
current_branch=$(git symbolic-ref --short HEAD)
if [ -z "$current_branch" ]; then
echo "You are not on a valid Git branch. Please switch to a branch and try again."
exit 1
fi
# Get the GitHub user and repository name
github_user_and_repo=$(git config --get remote.origin.url | sed 's/.*:\/\/github.com\///;s/.git$//')
# Get the pull request information
pr_info=$(hub pr list -f "%I %H%n" | grep $current_branch)
if [ -z "$pr_info" ]; then
open "https://github.com/$github_user_and_repo/compare/$current_branch"
else
# Get the PR number
pr_number=$(echo $pr_info | cut -d ' ' -f 1)
# Open the pull request in the browser
open "https://github.com/$github_user_and_repo/pull/$pr_number"
fi
5. Make the script executable by running:
chmod +x ~/.scripts/open-pr.sh
6. Add the following alias to your ~/.zshrc file:
alias openpr=""~/.scripts/open-pr.sh""
7. Reload your zsh configuration:
source ~/.zshrc
Now, whenever you want to open the current pull request in GitHub, simply type openpr in your terminal while in the repository folder. The script will open the pull request in your default browser if there is one or ask you to create one if there is no corresponding pull request.
Keep in mind that this script assumes you have proper authentication with GitHub (SSH or token). If not, you might need to set up your credentials with hub or git first.