- Published on Tuesday, January 23, 2024, last updated
Code Review Security Checklist
Table of Contents
- Principles of Secure Code review
- Understanding the OWASP Guidelines
- Integrating Security into the Software Development Life Cycle (SDLC)
- Secure Backend Code Review Checklist
- Secure Frontend Code Review Checklist
- Automated vs Manual Code Review
- List of Tools to Automate Code Review and Security Checks
- How About Human Code Reviews in Detecting Complex Vulnerabilities?
- Advanced Topics in Secure Code Review
- Threat Modeling in Code Review
- Dealing with Third-Party Libraries and Dependencies
- Continuous Integration and Continuous Deployment (CI/CD) in Secure Code Review
Security is a necessity, not just a feature. In this article, we'll explore the crucial role of code reviews in identifying and mitigating vulnerabilities. We'll delve into how to align with OWASP (Open Web Application Security Project) guidelines and effectively integrate these practices into the Software Development Life Cycle (SDLC). Additionally, we'll present a practical approach, complete with backend and frontend security checklists, to ensure your code stands up to the highest security standards. If you haven't read, I recommend looking at our .
Principles of Secure Code review
One of the foundational elements of a robust security posture in software development is the implementation of secure code review practices. Central to this is the understanding and application of the OWASP guidelines.
Understanding the OWASP Guidelines
The provides an extensive set of guidelines and best practices aimed at improving software security. The OWASP Code Review Guide is particularly significant for developers and security professionals. This guide encompasses a broad spectrum of security considerations, focusing on identifying common vulnerabilities and offering strategies for secure coding.
A pivotal resource within the OWASP framework is the OWASP Top 10, a regularly updated list highlighting the most critical security risks to web applications. This list serves as a starting point for developers to understand the most prevalent security threats and how to mitigate them effectively.
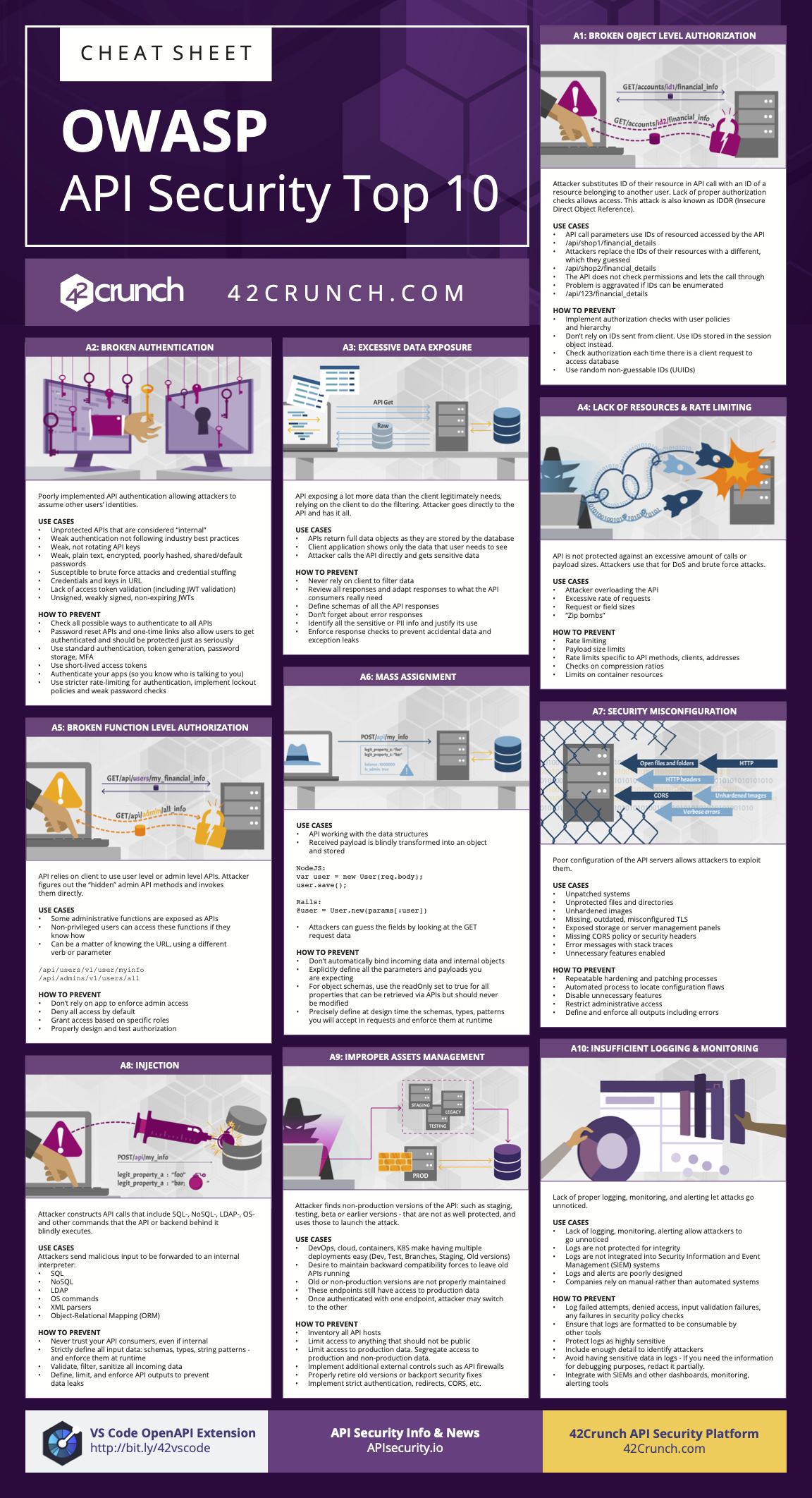
Integrating Security into the Software Development Life Cycle (SDLC)
Security in software development transcends beyond being a mere best practice; it's an integral necessity. It's not just the domain of developers but a collective responsibility shared across all roles involved in the software creation process. This holistic approach ensures that security permeates every aspect of the product's lifecycle.
- Designers: They can help focus on minimizing user errors that could lead to security breaches, ensuring intuitive and safe user interactions. By considering aspects like secure user authentication, data privacy, and access controls in their designs, they lay a foundation that supports robust security measures throughout the application.
- Product Managers: Responsible for defining the product's roadmap, they prioritize security features and requirements, making sure these considerations are embedded from the outset.
- Developers: Their role is critical in implementing secure coding practices, adhering to OWASP guidelines, and actively seeking to mitigate vulnerabilities during code development.
- Quality Assurance (QA): QA teams play a pivotal role in identifying security loopholes during testing phases, ensuring that the software not only functions as intended but also remains resilient against security breaches.
- Operations and Maintenance Teams: Post-deployment, these teams ensure ongoing security through monitoring, updating, and responding to new threats, thus maintaining the software's integrity over time.
By integrating security across these diverse roles in the SDLC, organizations cultivate a security-first culture. This approach not only enhances the security posture of the software applications but also ingrains a sense of shared responsibility towards security, making it a core aspect of the development ethos.
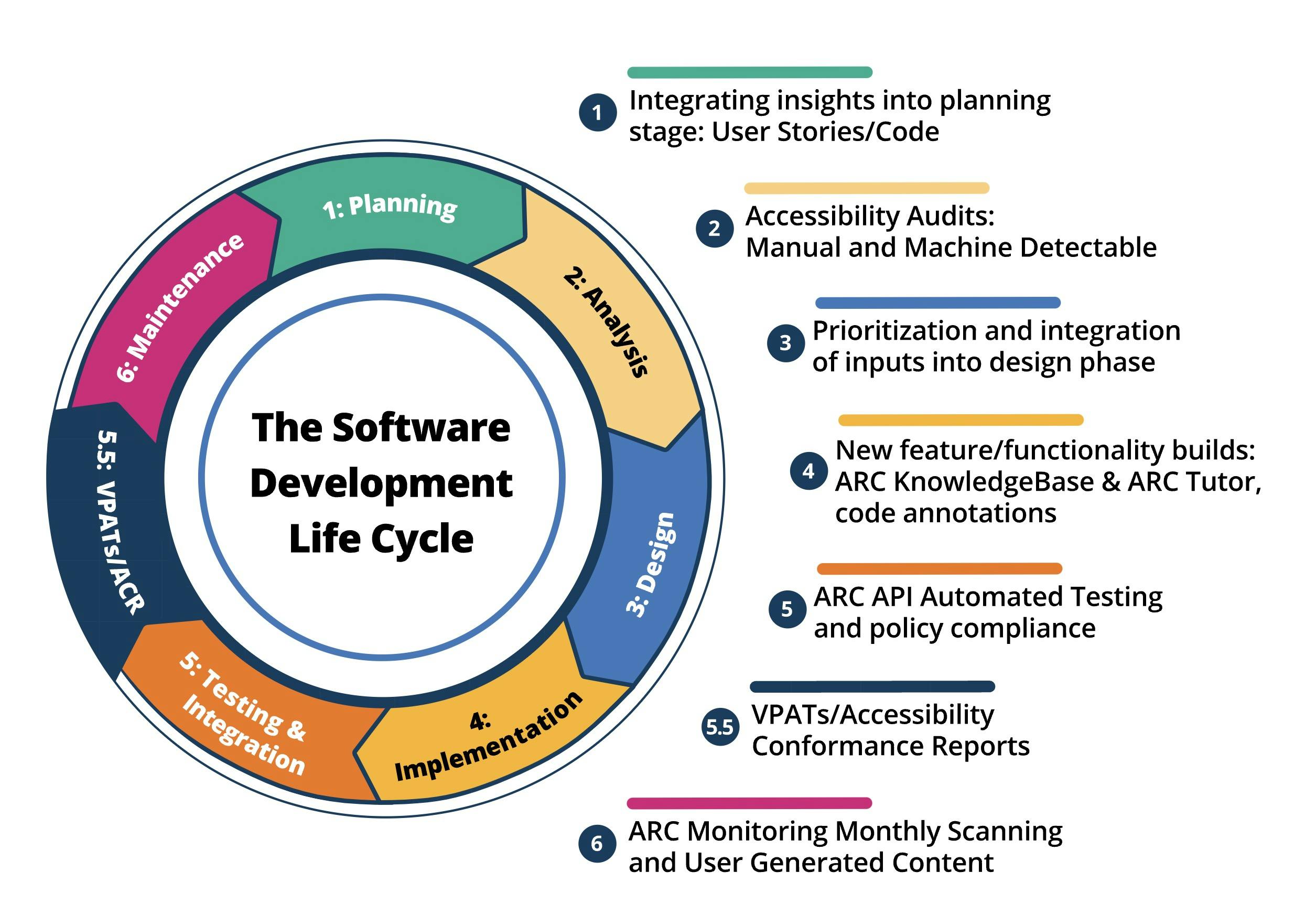
Security is collective responsibility shared across all roles involved in the software creation process
Secure Backend Code Review Checklist
The backend of any application is pivotal in managing data, business logic, and security. A secure backend is essential for safeguarding sensitive information and ensuring the overall security of the application. This checklist focuses on key areas in backend development where security is paramount.
## Secure Backend Code Review Checklist
**Security Vulnerabilities in Backend Development**
- [ ] Check for Injection Flaws: Prevent SQL, NoSQL, and command injections.
- [ ] Secure Authentication and Session Management: Implement robust mechanisms and secure session handling.
- [ ] Protect Sensitive Data: Use encryption and secure handling for passwords, tokens, and API keys.
**Common Security Pitfalls and How to Avoid Them**
- [ ] Avoid Hardcoded Credentials: Use environment variables or secure vaults instead of embedding credentials in code.
- [ ] Implement Adequate Logging and Monitoring: Set up comprehensive logging for detecting and addressing suspicious activities.
- [ ] Keep Security Settings Updated: Regularly review and update security configurations to current best practices.
**Reviewing Code for Critical Security Flaws**
- [ ] Conduct Code Analysis: Utilize static and dynamic analysis tools for identifying potential flaws.
- [ ] Audit Code Dependencies: Regularly update and review third-party libraries for vulnerabilities.
- [ ] Maintain High Code Quality: Adhere to good coding practices to prevent security issues.
**Secure Database Interaction and Data Management**
- [ ] Ensure Strict Data Validation: Implement input validation to avoid injection attacks.
- [ ] Apply the Principle of Least Privilege: Restrict database access to only essential operations.
- [ ] Encrypt Data: Secure data at rest and in transit with appropriate encryption methods.
**Review Completed**
- [ ] All checklist items verified and addressed.
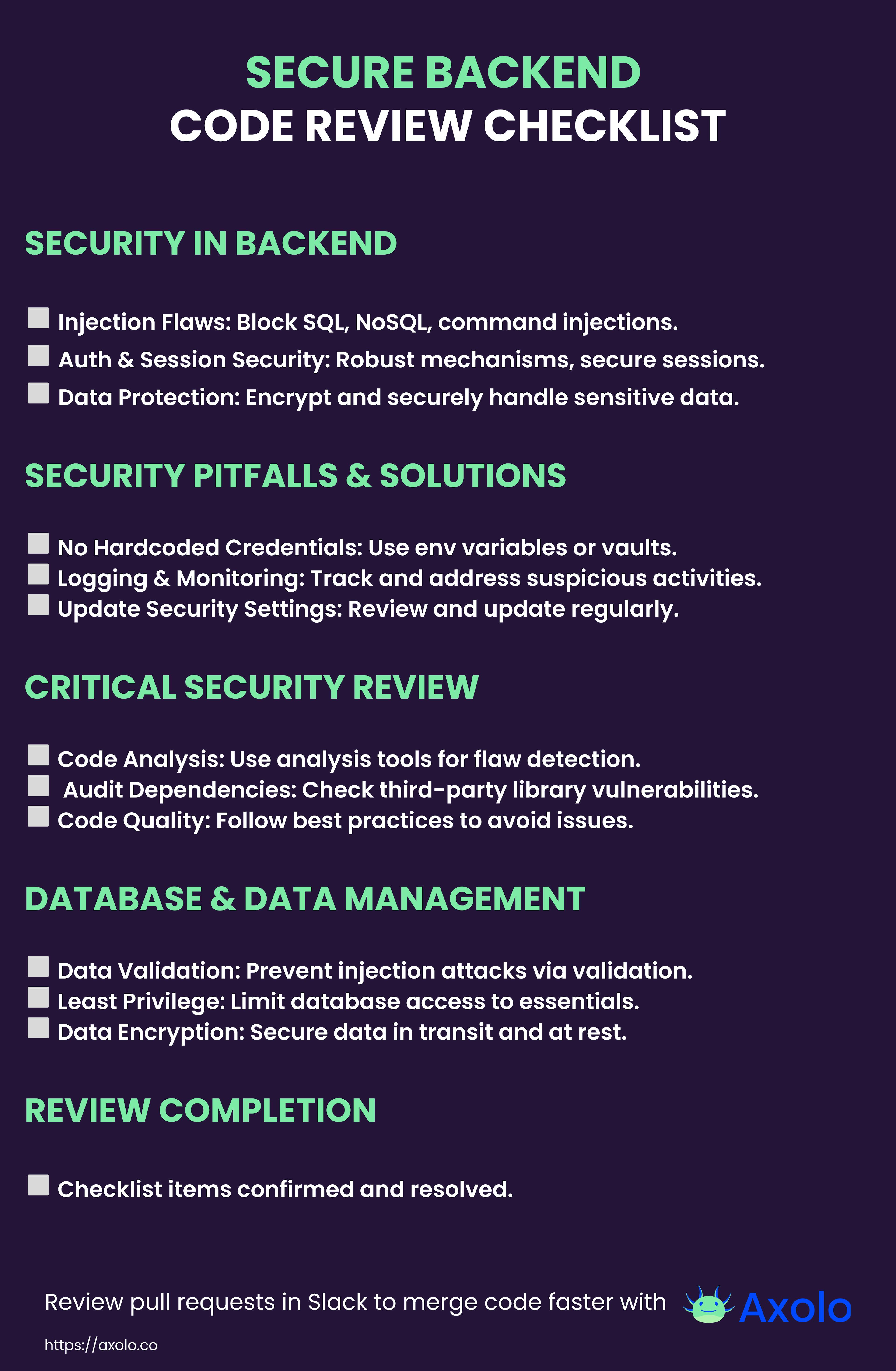
Share this image with your back end team!
Following these guidelines helps in creating a more secure backend environment, reducing the risk of security breaches and data compromises.
Secure Frontend Code Review Checklist
Frontend development faces unique security challenges, particularly with client-side processing. This checklist provides a concise guide to identifying and mitigating security risks in frontend code. It focuses on essential aspects like client-side risk mitigation, secure data handling, and robust user input validation. Use this checklist to enhance security in your frontend development process.
## Secure Frontend Code Review Checklist
**Security Considerations in Frontend Development**
- [ ] Implement Content Security Policy (CSP): Protect against cross-site scripting and other code injection attacks.
- [ ] Secure Communication: Use HTTPS to encrypt data in transit.
- [ ] Minimize Exposed Data: Limit sensitive data exposure in client-side applications.
**Identifying and Mitigating Client-Side Risks**
- [ ] Cross-Site Scripting (XSS) Protection: Validate and sanitize user input to prevent XSS attacks.
- [ ] Cross-Site Request Forgery (CSRF) Prevention: Implement anti-CSRF tokens in forms.
- [ ] Clickjacking Protection: Use X-Frame-Options to prevent embedding of site content in iframes.
**Ensuring Secure Data Transmission and Storage**
- [ ] Use Secure APIs for Data Transmission: Ensure APIs used for data transfer employ secure protocols.
- [ ] Avoid Storing Sensitive Data in Local Storage: Use secure methods for handling sensitive user data.
- [ ] Implement Proper Session Management: Manage sessions securely to protect user data.
**Handling User Input and Validation**
- [ ] Validate Input on Client and Server Side: Ensure all user input is validated both on the frontend and backend.
- [ ] Sanitize User Input: Prevent injection attacks by sanitizing user inputs.
- [ ] Use Framework-Specific Protection: Employ security features provided by your frontend framework for input validation.
**Review Completed**
- [ ] All checklist items verified and addressed.
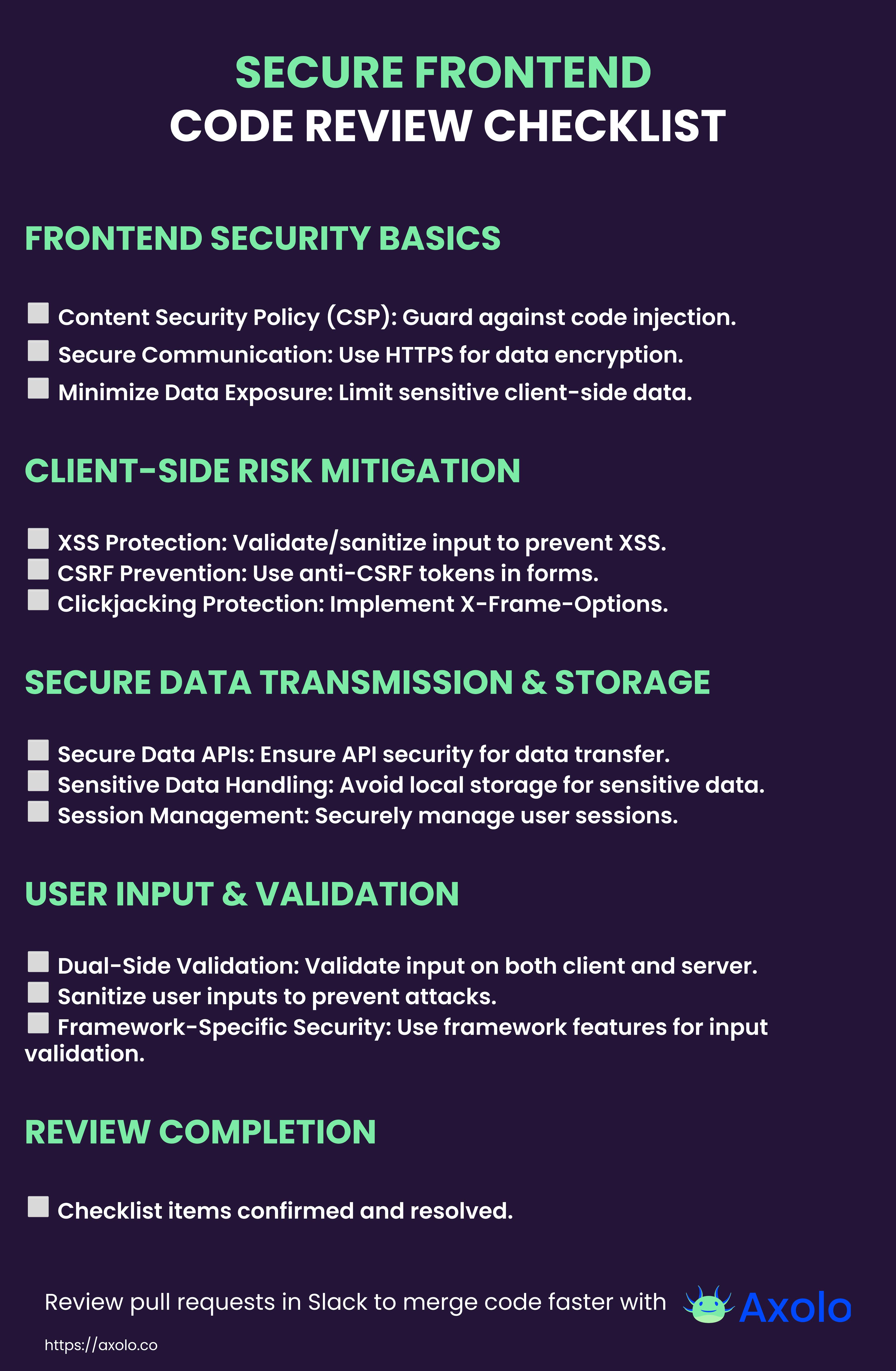
Share this image with your front end team!
Automated vs Manual Code Review
In code reviews, both automated and manual approaches play critical roles. Automated tools can significantly speed up the review process and detect common vulnerabilities, while manual reviews are essential for uncovering more complex issues that automated tools might miss.
List of Tools to Automate Code Review and Security Checks
Tool Name | Description | Pros | Cons | Price |
---|---|---|---|---|
SonarQube | Continuous inspection of code quality to detect bugs and vulnerabilities. | Comprehensive analysis, supports multiple languages. | Can be complex to set up. | Free Community Edition; Paid plans available. |
ESLint | Pluggable JavaScript linter that identifies and reports on patterns. | Highly configurable, great for JavaScript. | Mainly for JavaScript; requires configuration. | Free. |
Veracode | Automated security testing for application codebases. | Wide language support, integrates with CI/CD. | Can be costly for small teams. | Pricing upon request. |
Checkmarx | Static Application Security Testing (SAST) tool for security vulnerability scanning. | Comprehensive SAST tool, good CI integration. | Expensive for smaller projects. | Pricing upon request. |
Fortify | Static and dynamic security testing of applications. | Robust feature set, supports a wide range of languages. | High cost and complexity. | Pricing upon request. |
CodeClimate | Analyzes source code for performance issues, vulnerabilities, and complex patterns. | Easy to integrate, good for code quality tracking. | May lack depth in security analysis. | Free for open source; Paid plans for private repos. |
How About Human Code Reviews in Detecting Complex Vulnerabilities?
While automated tools are efficient for scanning large codebases and identifying standard vulnerabilities, they often lack the nuanced understanding that comes from human expertise. Manual code review is crucial for:
- Understanding Context: Human reviewers can interpret the context and intent behind the code, which is vital for identifying complex security issues.
- Business Logic Errors: Manual review is better suited for detecting flaws in business logic, which automated tools may overlook.
- Code Quality and Maintainability: Human reviewers can provide insights into code readability, maintainability, and adherence to best practices.
Combining both automated tools and manual review processes ensures a comprehensive approach to code review, enhancing the overall security and quality of the software.
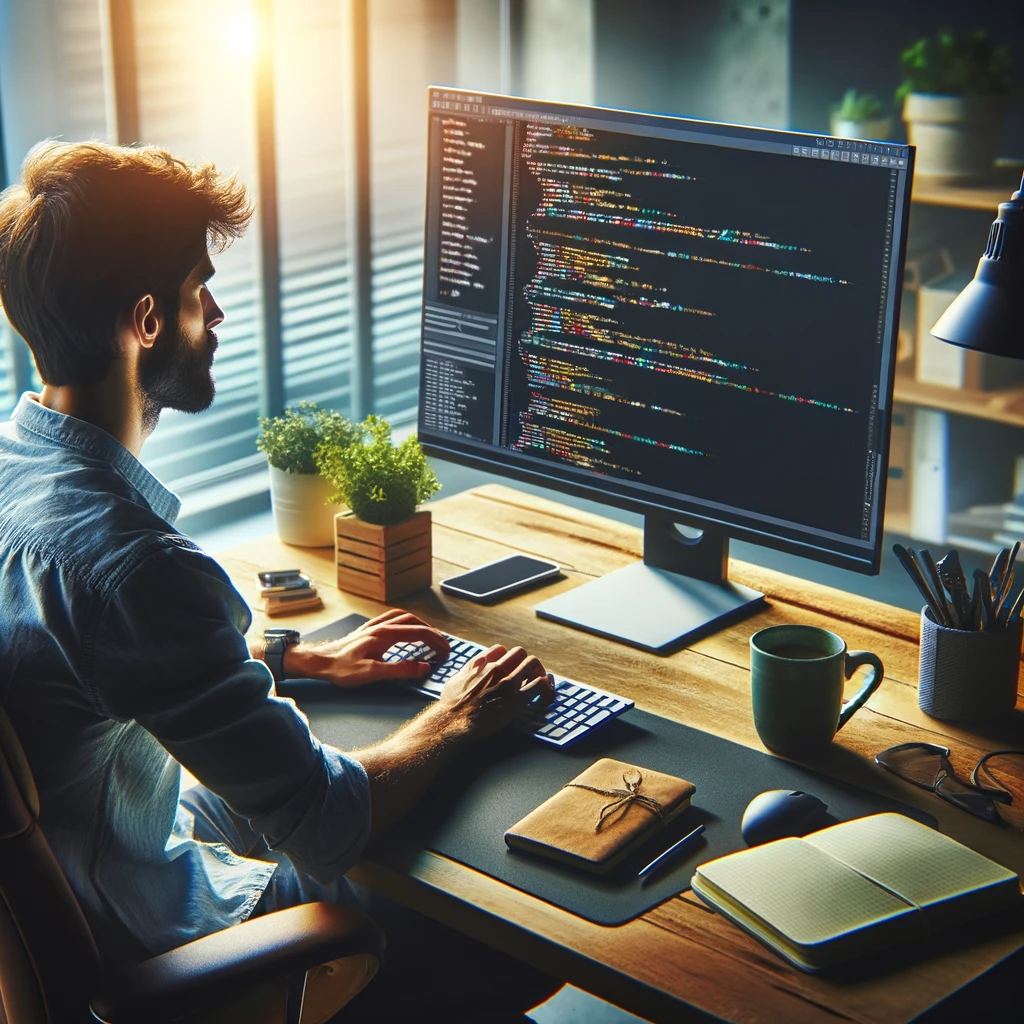
Human reviewers can interpret the context and intent behind the code, which is vital for identifying complex security issues.
Advanced Topics in Secure Code Review
As code review practices evolve, several advanced topics emerge as crucial for maintaining robust security in software development. Understanding these advanced concepts can significantly enhance the effectiveness of your code review process.
Threat Modeling in Code Review
- Concept: Threat modeling involves identifying potential security threats and vulnerabilities in the early stages of development and during the code review process.
- Application: Incorporate threat modeling to anticipate how attackers might compromise your software and use this insight to inform code reviews.
- Benefits: Helps in proactively addressing security issues and strengthens the software against potential attacks.
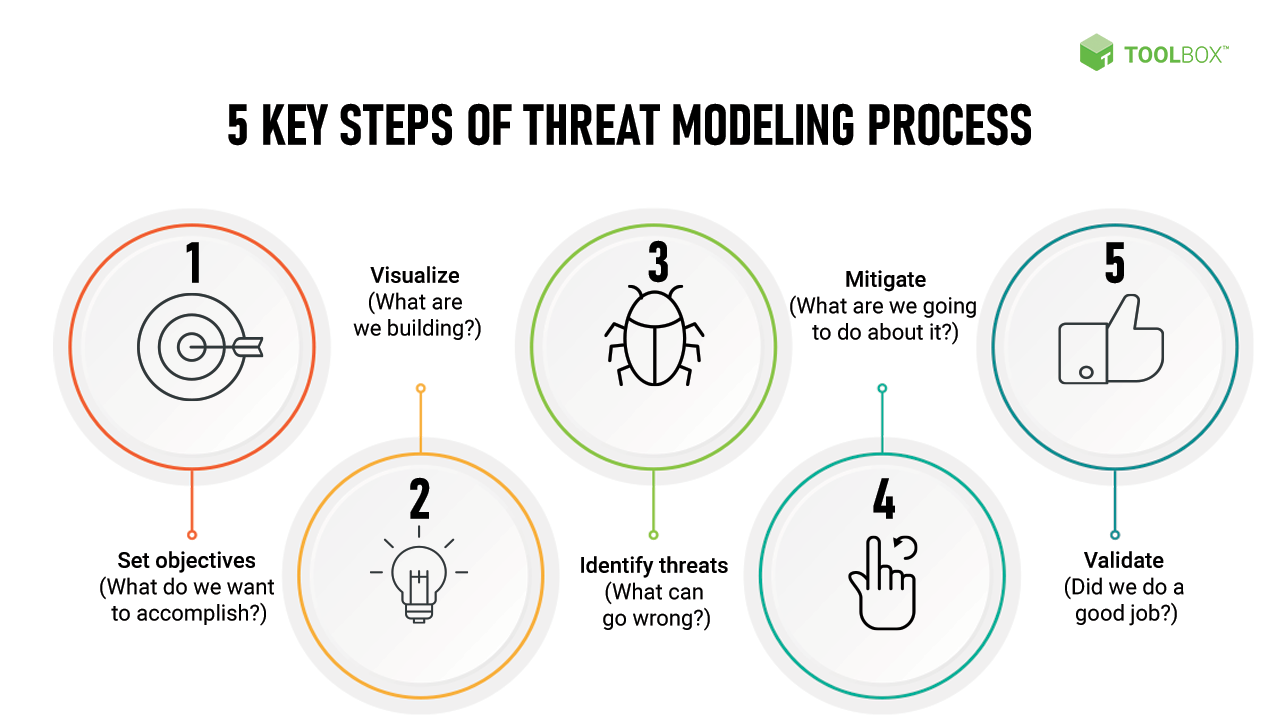
Axolo is a Slack app to help techteams review pull request seamlessly
Dealing with Third-Party Libraries and Dependencies
βThird party threats are one of the most common security threats in software development.β
Third party threats are one of the most common security threats in software development. When using a third party library make your research, is it a library that is being used by many people? When was the last update made to this project? Do they have automated tests?
- Best Practices: Regularly audit and update third-party libraries. Use tools like to identify known vulnerabilities.
- Risk Management: Understand the security posture of third-party components and weigh the risks versus benefits.
Continuous Integration and Continuous Deployment (CI/CD) in Secure Code Review
- Integration with CI/CD: Automate security checks as part of the CI/CD pipeline to ensure continuous assessment of the code.
- Tools and Techniques: Utilize tools like with security plugins or for automated testing and code analysis. We've made you can find.
- Continuous Security: Implementing secure code review practices in CI/CD allows for ongoing security verification throughout the development process.
This highlight the need for a proactive approach to a secure code review process, ensuring that security is an integral part of the development process rather than an afterthought.