- Published on Wednesday, July 3, 2024, last updated
Ultimate Guide to Git Commit Message Templates: Best Practices and Examples
Want to enhance your team’s code review process with clear, consistent Git commit messages? This guide walks you through best practices, real-life examples, and advanced techniques to keep your commit history easy to understand and collaborate on.
Table of Contents
- TLDR: Git Commit Message template
- Introduction
- Importance of Clear Git Commit Messages
- Git Commit Messages Checklist
- Part 1: Understanding Git Commit Messages
- Part 2: Structure of a Git Commit Message
- Part 3: Writing Effective Git Commit Messages
- Part 4: Git Commit Message Best Practices
- Part 5: Git Commit Message Best Practices Examples
- How to Set Up a Git Commit Message Template
- Part 6: Automating Git Commit Messages
- Part 7: Common Pitfalls and How to Avoid Them
- Part 8: Git Commit Message Conventions
- Tools and Resources for Better Commit Messaging
- Part 9: Advanced Git Commit Message Techniques
- Conclusion
- Appendix
TLDR: Git Commit Message template
Use the following examples to craft clear and effective Git commit messages for various scenarios:
# GITHUB COMMIT MESSAGE TEMPLATE EXAMPLES
## Commit message with description and breaking change footer
feat: allow provided config object to extend other configs
BREAKING CHANGE: 'extends' key in config file is now used for extending other config files.
## Commit message with ! to draw attention to a breaking change
feat!: send an email to the customer when a product is shipped
## Commit message with scope and ! to draw attention to a breaking change
feat(api)!: send an email to the customer when a product is shipped
## Commit message with both ! and BREAKING CHANGE footer
chore!: drop support for Node 6
BREAKING CHANGE: use JavaScript features not available in Node 6.
## Commit message with no body
docs: correct spelling of CHANGELOG
## Commit message with scope
feat(lang): add Polish language
## Commit message with multi-paragraph body and multiple footers
fix: prevent racing of requests
Introduce a request id and a reference to latest request. Dismiss
incoming responses other than from the latest request.
Remove timeouts which were used to mitigate the racing issue but are
obsolete now.
Reviewed-by: Z
Refs: #123
## Commit message for a minor bug fix
fix(ui): resolve issue with dropdown menu alignment
The dropdown menu was misaligned on the profile page due to CSS conflicts.
Updated the styles to ensure proper alignment across different screen sizes.
Fixes issue #789.
## Commit message for adding a new test
test: add unit tests for user authentication module
Added unit tests for the user authentication module to cover login, registration, and token verification functionalities.
Ensured all tests pass with the new authentication logic.
Refs: #456
## Commit message for a configuration change
chore(config): update ESLint configuration to support latest standards
Updated the ESLint configuration to support the latest JavaScript standards and best practices.
Included new rules for code formatting and improved error detection.
Reviewed-by: A, B
Introduction
Importance of Clear Git Commit Messages
Clear, well-structured Git commit messages play a crucial role in the development process. They offer context for every change, making project management and collaboration more streamlined. By explaining the reasons behind updates, these messages prevent confusion and help teams maintain a solid version history.
Git Commit Messages Checklist
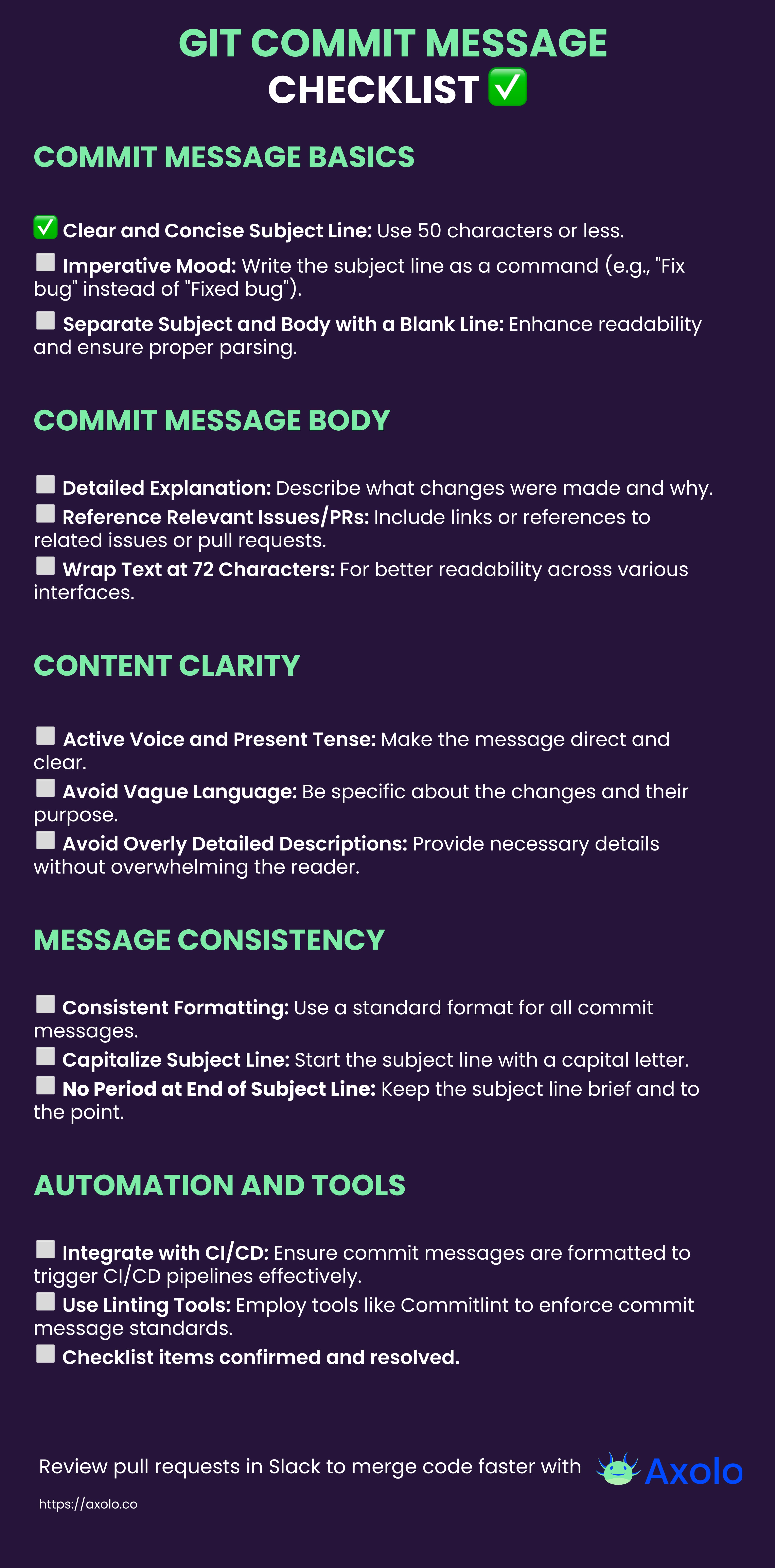
Enhancing Project Management and Collaboration
Good commit messages can significantly boost teamwork and project organization:
- Clarity and Purpose: Each message quickly tells others what has changed and why it was needed. This reduces onboarding times for new developers and helps existing contributors revisit the codebase with minimal friction.
- Simplifying Code Reviews: A well-written message gives reviewers insight into the scope and objective of a change, making it easier to focus on the code itself.
- Better Teamwork: Detailed commit notes improve communication by illustrating how everyone’s work fits together.
For more detailed guidelines, refer to Git Commit Guidelines.
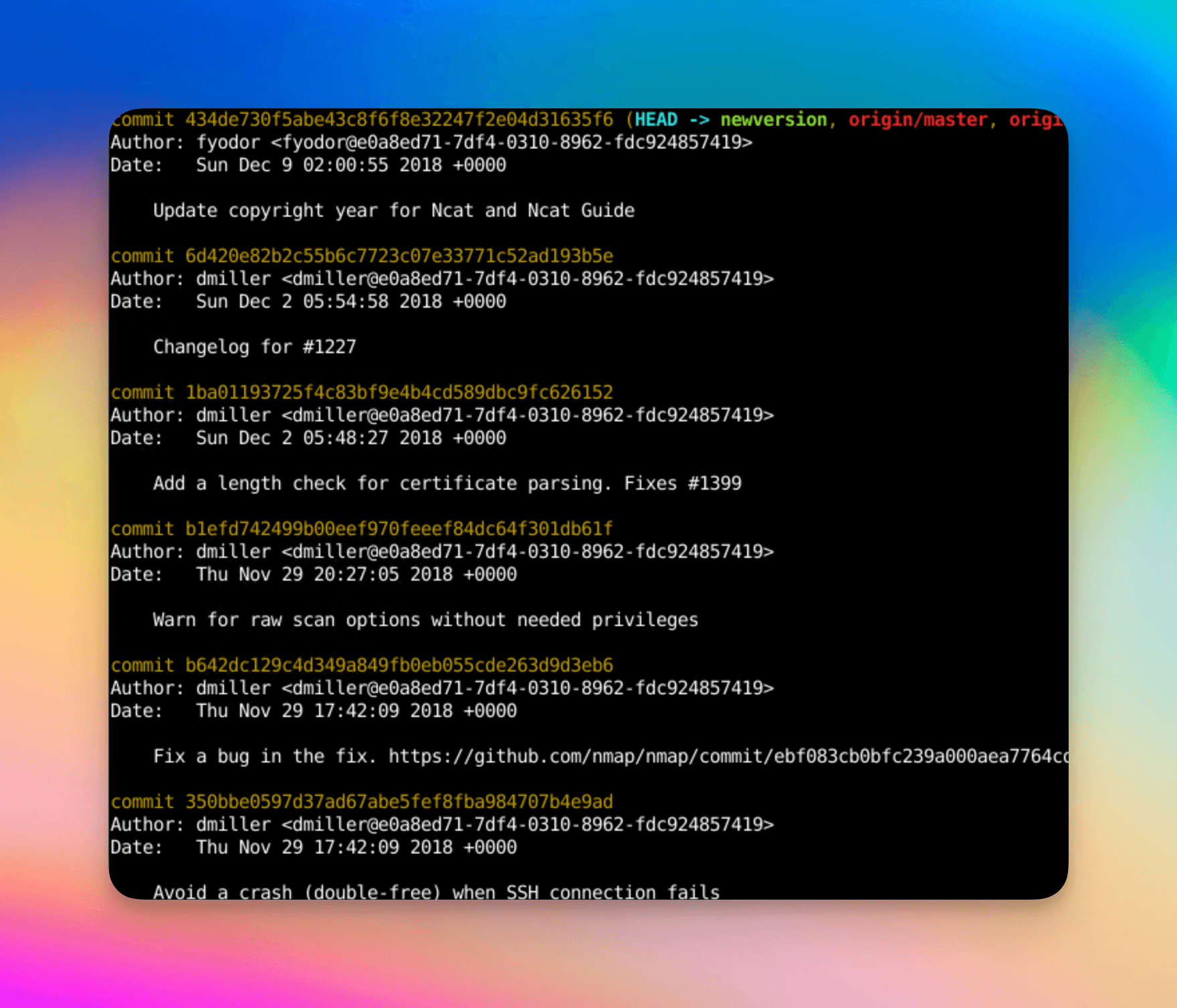
If you type Git Log in your Git Repository in your terminal you will see a Git history that looks similar to this!
Benefits for Code Tracking and Version Control
Commit messages also act as your project’s historical breadcrumbs:
- Traceability: It’s easier to backtrack changes and figure out which commit introduced an issue.
- Simple History Navigation: Clear messages let you search for specific fixes or features when the repository grows.
- Smooth Automation: Tools like continuous integration or release pipelines often rely on commit messages to trigger certain actions or generate notes.
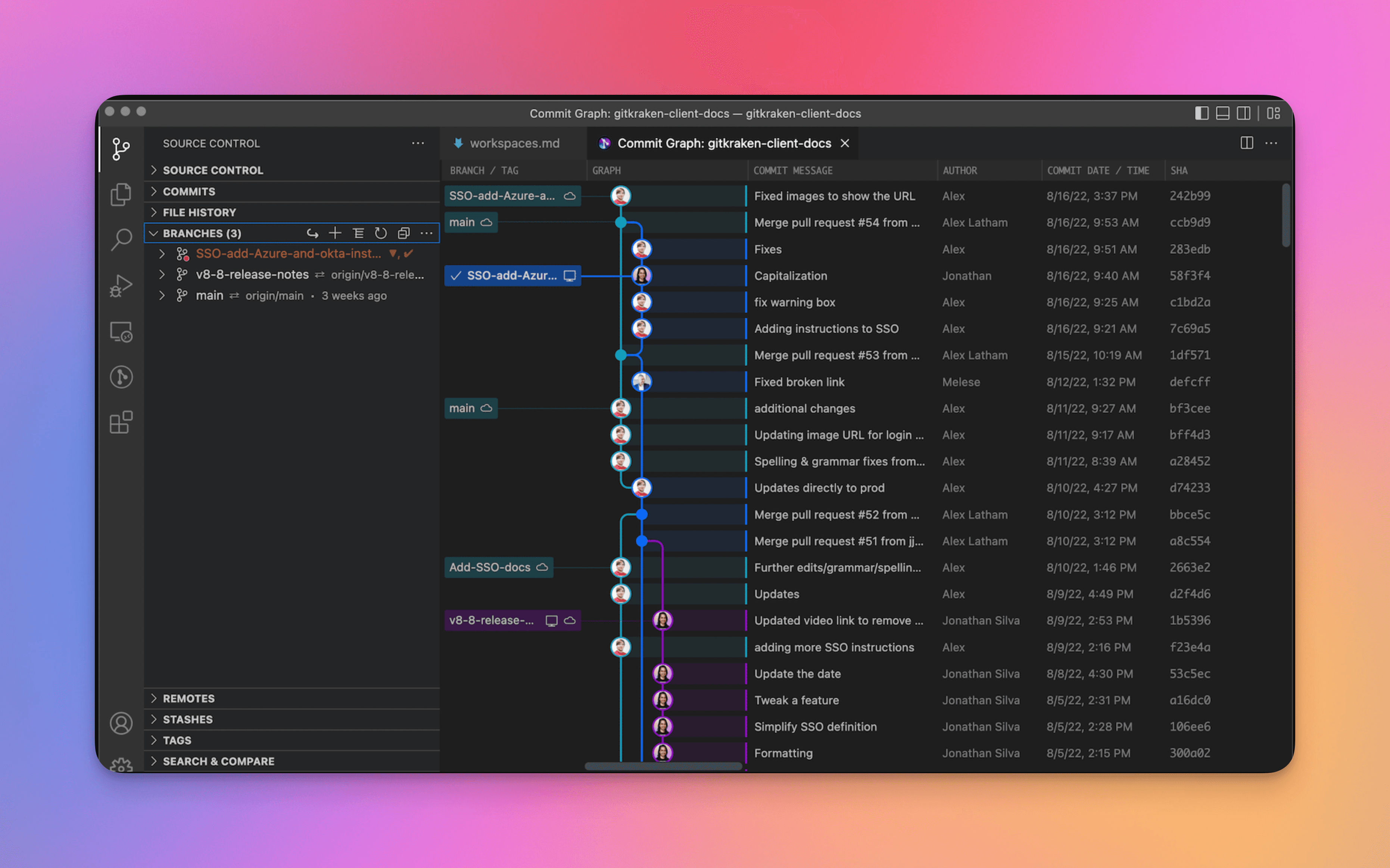
Here is a Git History using GitLens in VS Code. It clearly shows which modifications were made by whom, with descriptive messages. This can be highly beneficial when debugging.
Check out Git Documentation on Commit Messages for more information.
Following best practices in writing commit messages helps keep the repository organized, fosters clearer communication, and improves overall productivity for the entire team.
Part 1: Understanding Git Commit Messages
What is a Git Commit Message?
A Git commit message is a short note that goes with each commit in a Git repository. It shows what was changed and explains why those changes were made, serving as an important piece of documentation in your version control process.
Definition and Purpose: Every commit message clarifies the motivation behind a particular change, helping current and future developers understand the reasoning behind edits when they look back at the project’s history. Atlassian Git Tutorials
Anatomy of a Commit Message: Generally, a well-written commit message has three parts: a subject line, a body, and a footer. The subject line sums up the changes, the body dives into the details, and the footer can include extra data like issue IDs or references. Conventional Commits
Providing this level of detail is particularly helpful when dealing with complex projects, since it clarifies why certain paths were taken. Maintaining informative commit messages makes your version history a valuable resource for the entire team.
Why Git Commit Messages Matter
Beyond day-to-day coding tasks, commit messages play a significant role in the health of a software project:
- Streamlining Code Reviews: Explaining the scope and purpose of changes helps reviewers provide better feedback.
- Easier Debugging and Troubleshooting: A well-documented commit history accelerates the process of finding when and how bugs were introduced.
- Project Onboarding: New contributors gain insights into the evolution and decision-making behind the code.
- Workflow Automation: Many CI/CD pipelines rely on commit messages for actions like generating changelogs or deploying features.
By consistently writing informative commit messages, developers keep the repository’s history an asset rather than a hindrance.
Part 2: Structure of a Git Commit Message
Subject Line
Each commit message starts with a subject line that briefly captures the essence of your changes. It gives teammates a quick snapshot of the commit’s purpose.
- Concise Summary (50 characters max): Keep your subject line short—ideally no more than 50 characters—so it’s easy to read in various Git tools. How to Write a Git Commit Message
Body
The body of your commit message can include technical details, design reasons, or other relevant background that shaped the changes you made. Writing Good Commit Messages
Referencing Issues or Pull Requests: If possible, mention any related issues or PRs to provide additional context and keep the broader discussion linked. Referencing Issues in Commits
Paragraph Breaks: Use distinct paragraphs for different points to maintain clarity. This makes it easier for others to scan and understand your message. Commit Message Guidelines
Footer
In the footer, you can list any relevant metadata:
(See also Sign-off in Commit Messages for more details.)
- Signed-off-by: Some teams require a “Signed-off-by” line in the footer. This is particularly important for projects adhering to the Developer Certificate of Origin (DCO). Git Documentation on Sign-offs
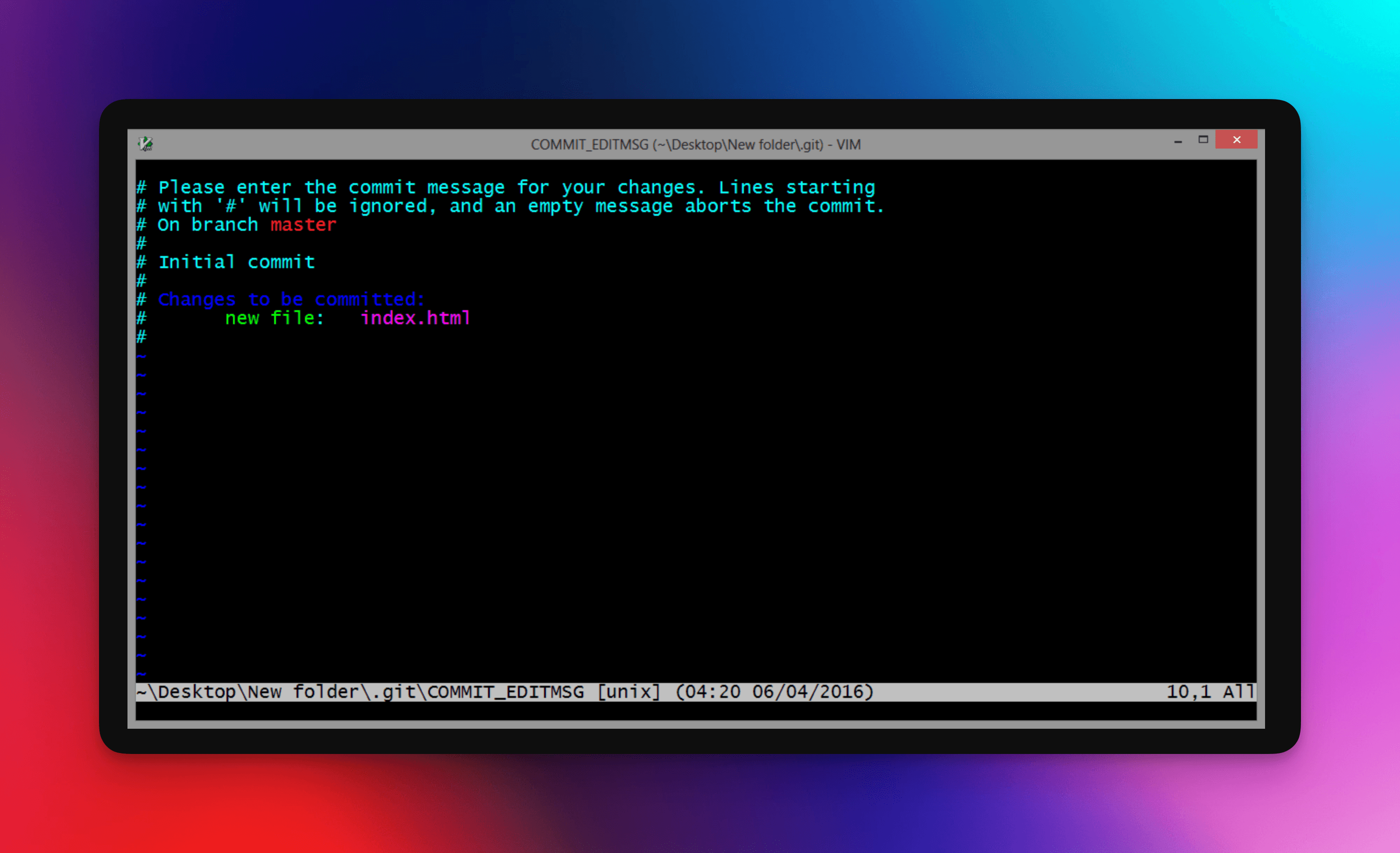
Git committing using Vimium
Part 3: Writing Effective Git Commit Messages
Tips for Crafting Clear and Concise Messages
Clear and concise commit messages can make your codebase significantly easier to maintain and review. Below are some suggestions:
- Be Specific: Avoid vague text. Summarize the change precisely and mention why it was needed. Effective Commit Messages
- Active Voice & Present Tense: Opt for a direct, present-tense style (e.g., “Fix bug” rather than “Fixed bug”). It’s more straightforward and common in version control logs. Active Voice in Commit Messages
The Imperative Mood
Many teams recommend writing commit messages in the imperative mood. It keeps messages consistent and clearly states the action the commit will take when applied.
- Why Imperative Mood Matters: Short commands like “Add” or “Fix” set an easy-to-read standard. Imperative Mood in Git Commit Messages
- Examples: “Add new feature,” “Fix layout bug,” etc. Sample Commit Messages
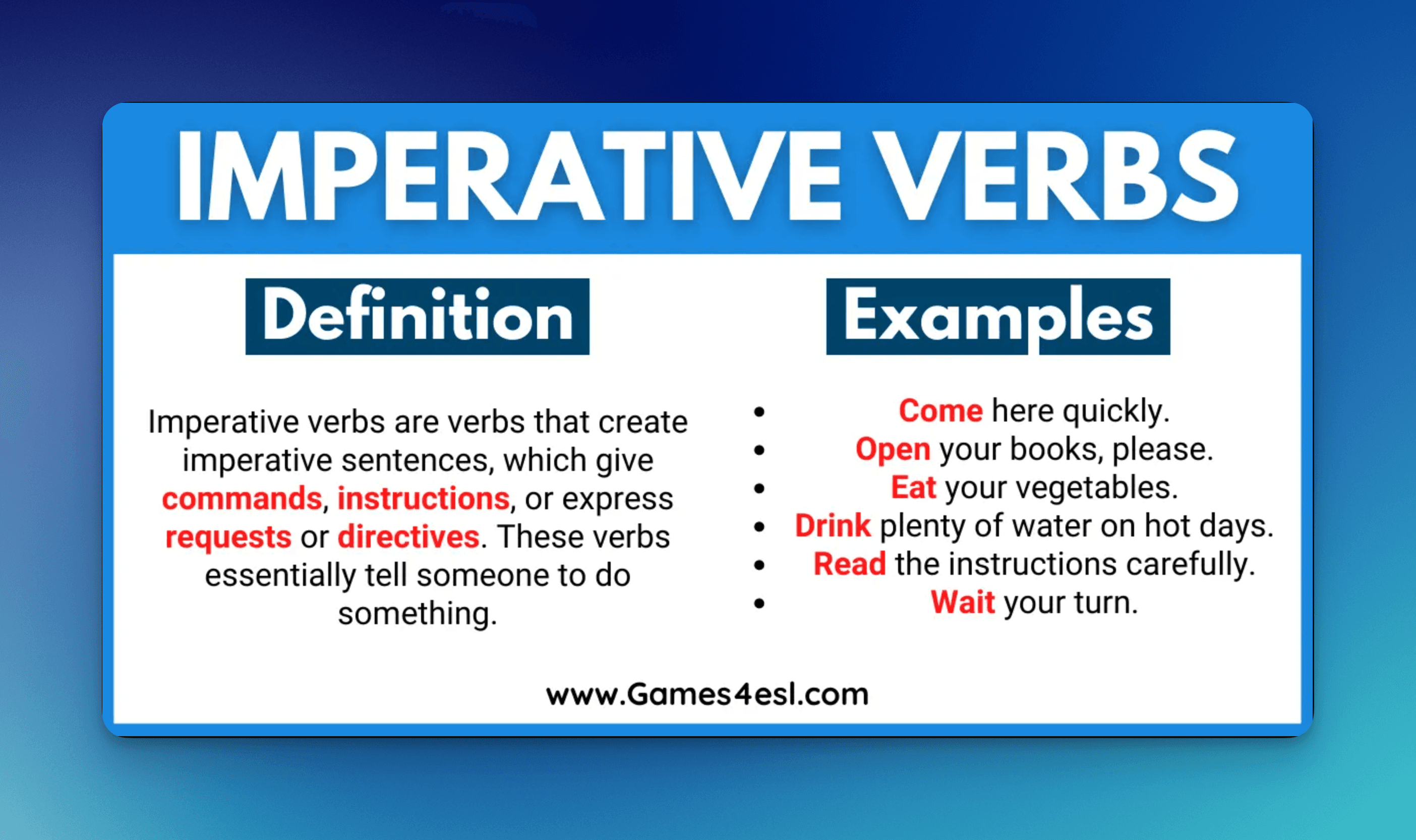
Using Markdown for Formatting
Markdown is a straightforward markup language that helps format commit messages, making them more visually appealing.
- Bullet Points & Code Blocks: Bullets can break down changes into clear steps, while code blocks showcase snippets cleanly. Markdown Guide
- Improved Formatting: Adopting Markdown features can improve how others read and digest your commit details. GitHub Flavored Markdown
Part 4: Git Commit Message Best Practices
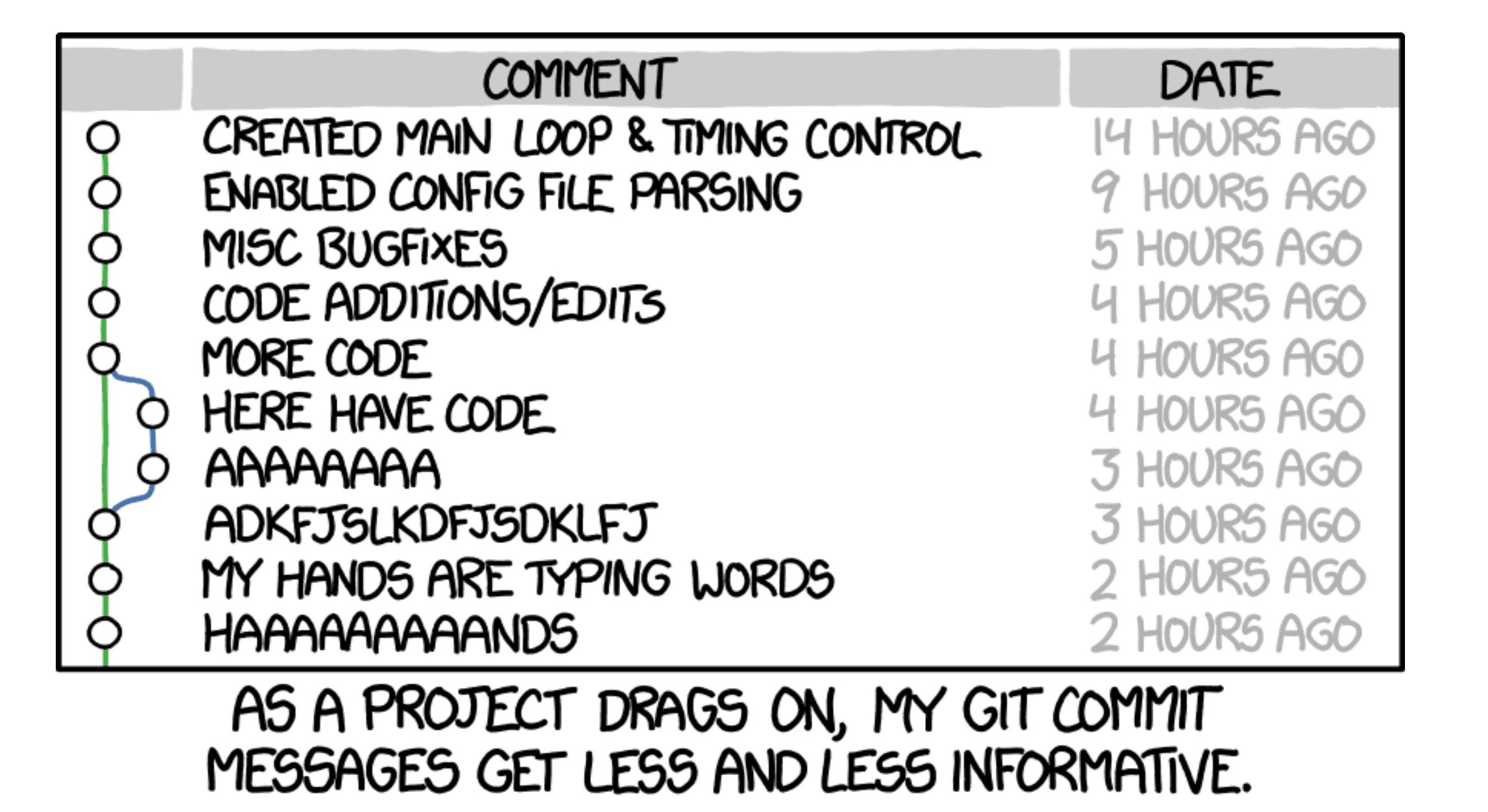
Crafting strong commit messages blends clarity, brevity, and structure. Below are several tried-and-true best practices.
Keep Messages Short but Descriptive
Aim for a concise message that still conveys the essential changes. Many teams recommend a subject line of up to 50 characters.
Separate Subject and Body with a Blank Line
Placing a blank line between the subject and the body ensures better readability and compatibility with various Git tools.
Use the Body to Explain the “What” and “Why,” Not the “How”
Focus on the reason behind the change rather than the implementation details. The code itself shows how something is done.
Reference Issues and Pull Requests
Linking an issue or PR number helps with traceability, so everyone knows the broader discussion.
Capitalize the Subject Line
This simple convention makes each commit message look cleaner.
Avoid Ending the Subject with a Period
It’s unnecessary, and skipping it maintains a uniform style.
Wrap the Body at 72 Characters
A 72-character line width is typical, keeping messages neatly formatted in various tools.
Examples of Good vs. Bad Commit Messages
Good:
Subject: Fix null pointer exception in login Body: Resolved a null pointer error occurring when users log in without credentials. Added validation to check for null values before processing requests. Fixes issue #123.
Bad:
Subject: Fix bug Body: fixed the bug
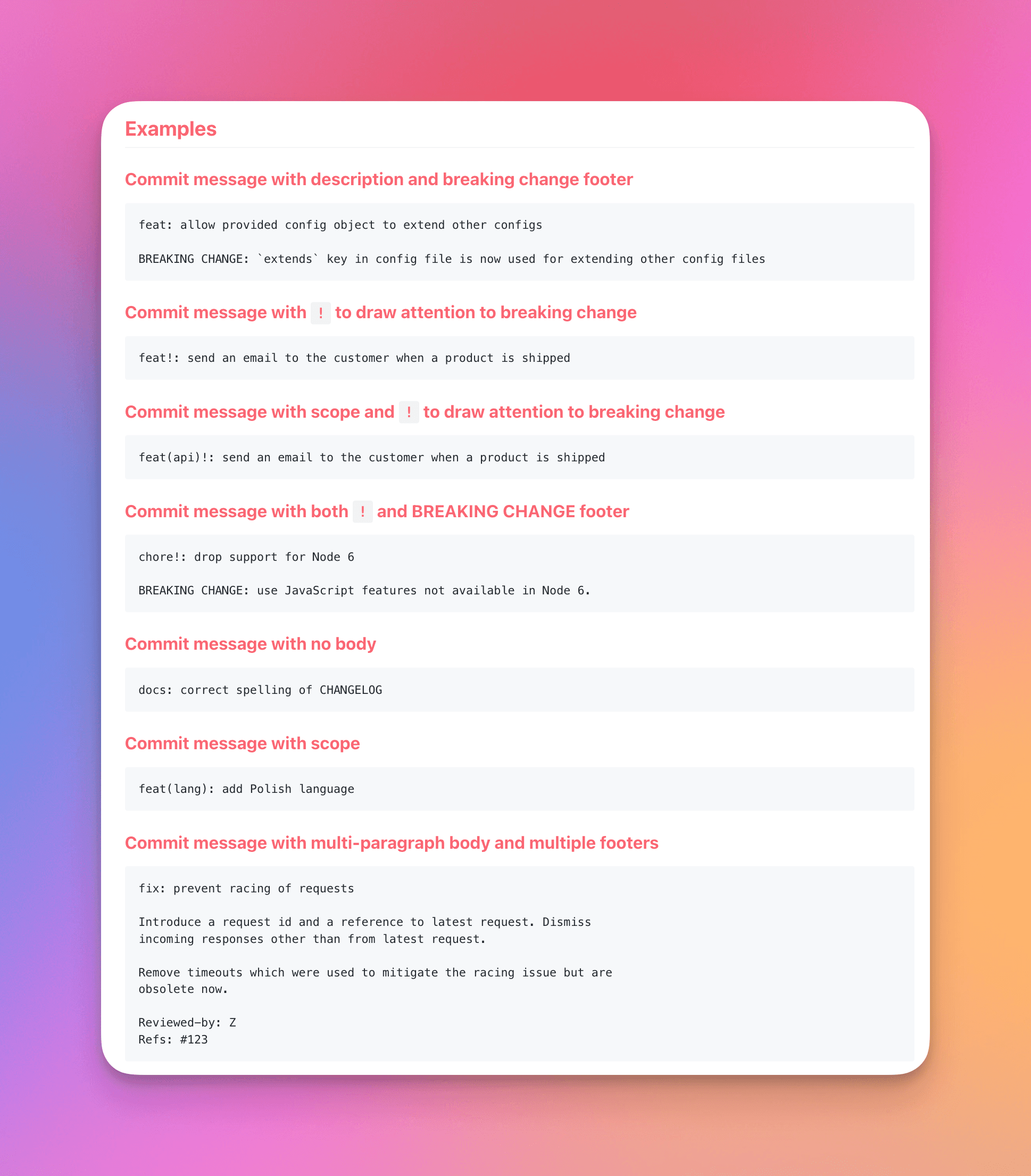
Part 5: Git Commit Message Best Practices Examples
To demonstrate these guidelines, here are some well-crafted commit messages:
Adding a New Feature
Subject: Add user authentication feature Body: Implemented user authentication with JWT. Introduced login and registration endpoints. Updated frontend components to handle tokens.
Fixing a Bug
Subject: Fix null pointer exception in login Body: Resolved a null pointer issue triggered by empty credentials. Added validation to ensure user input fields are not null. Fixes #123.
Updating Documentation
Subject: Update README with setup instructions Body: Added details for local environment setup. Clarified database configuration steps.
Refactoring Code
Subject: Refactor user service to improve maintainability Body: Broke down large methods into smaller functions. Added unit tests to ensure correctness post-refactor.
Improving Performance
Subject: Optimize database queries for user endpoints Body: Introduced indexes to speed up user lookups. Reduced query complexity in the user service. Gains of up to 30% in local benchmarks.
Axolo User Experiences
2480+ developers online
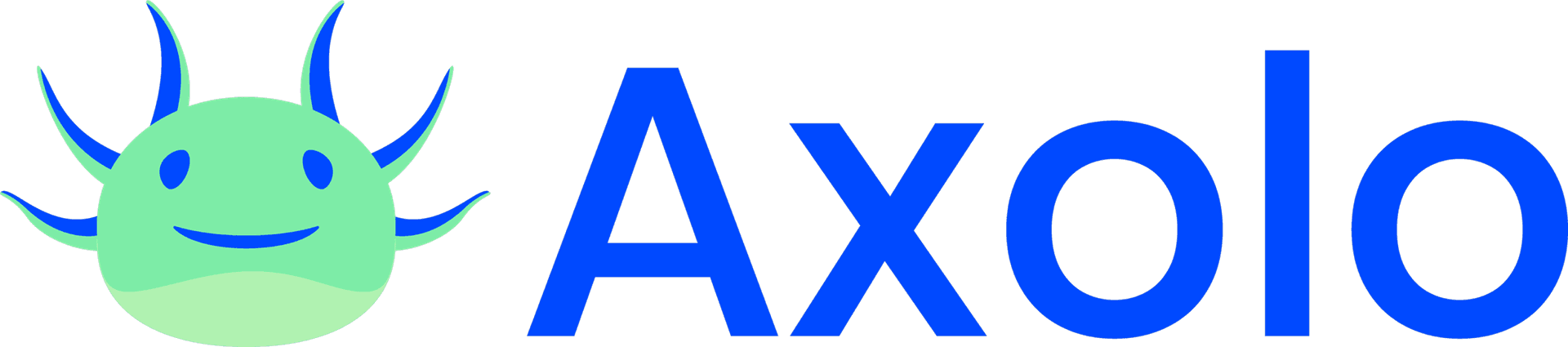
How to Set Up a Git Commit Message Template
Using a template helps your team consistently follow a git commit message format, preventing confusion and making it simpler to update commit message guidelines if needed.
Create a Template File
Make a file called
commit-template.txt
in your project.Include a structure, for example:
Subject (50 chars max): Body (why this change? details about changes made): Footer (issue references, co-authors, etc.):
Configure Git to Use the Template
- Set the template globally:
git config --global commit.template path/to/commit-template.txt
- Or just for one project:
git config commit.template path/to/commit-template.txt
- Set the template globally:
Validate the Template
- Test a commit to ensure you’re prompted with the template.
- If your team regularly discusses pull request vs merge request, you might also provide guidance in the template about referencing these processes.
A commit message template is handy for teams practicing semantic commits or any strict git commit message convention. It also encourages writing good commit messages by reminding everyone about critical elements like referencing tickets or giving a clear git commit description. If inconsistencies arise, prompt teammates to change commit message strategies or fix commit message mistakes as necessary.
Tools with a git view commit message function let you review your template-based messages before pushing them. Whenever your style changes, it’s easy to update commit message formatting by refining the template.
Part 6: Automating Git Commit Messages
Automation tools help maintain consistent github commit messages standards, so every commit message remains clear and uniform.
Tools for Enforcing Commit Message Standards
Commitlint: Checks if messages follow certain formats (like conventional commits). Commitlint Documentation
Screenshot of how Commitlint works
Husky: Runs Git hooks easily, often combined with Commitlint to validate messages. Husky Documentation
Git Hooks: Custom scripts triggered at specific points in the Git workflow (e.g., pre-commit, commit-msg). Git Hooks Documentation
Setting Up Commit Message Templates
- Creating a Commit Template in Git:
Using Git Commit Templates
- Examples and Customization:
Commit Message Template Examples
Part 7: Common Pitfalls and How to Avoid Them
Even with guidelines, it’s easy to slip into bad habits. Here are some frequent pitfalls:
Vague Commit Messages
Phrases like “update code” or “fix stuff” don’t explain anything. Be precise about the change and its motivation.
Overly Detailed Commit Messages
On the flip side, massive blocks of text can obscure the main idea. Strike a balance between clarity and brevity.
Ignoring the Subject Line
Always give a clear, concise subject line. This is the first thing people see and should summarize the commit at a glance.
By avoiding these common errors, you’ll maintain a clean, navigable history.
Part 8: Git Commit Message Conventions
Conventions add clarity to your project’s commit history, simplifying how changes are categorized and understood.
Conventional Commits
A lightweight format specifying commit types (e.g., feat
, fix
, docs
) and encouraging structured messages. Conventional Commits
Semantic Commit Messages
Align your commit messages with semantic commits. This approach automates version bumps when you release. Semantic Versioning
Customizing Commit Message Guidelines for Your Team
Adopt existing formats or tailor them to your workflow. The goal is to keep messages uniform and easy to parse. A commit convention can unify everyone’s approach.
Tools and Resources for Better Commit Messaging
Besides automating and standardizing your github commit message best practices, here are additional tools and references:
Axolo for code review
code review
Use Axolo to open Slack channels for each PR, keeping commit and update commit message discussions centralized.GitHub Code Review Best Practices
Read up on github code review best practices to see how commit messages fit into broader development cycles.Pull Request Guides
If your team debates pull request vs merge request or wonders what is a pull request, aligning commit conventions with your PR workflow ensures clarity. This also helps optimize your github pull request workflow.GitHub Templates
A github pull request template works in tandem with standardized github commit messages.GitHub Draft PR
A github draft pr lets you experiment before finalizing commits.GitHub Actions on Pull Request
Use github actions on pull request to automate tasks like linting or commit checks.
Embracing these resources encourages a mindset of continuous improvement, so you can fine-tune or change commit message approaches as your project’s needs evolve.
Part 9: Advanced Git Commit Message Techniques
For bigger teams or open source initiatives, advanced methods can further enhance clarity and collaboration.
Using Emojis in Commit Messages
Adding emojis can make commit messages more visually distinctive, hinting at their nature at a glance. Using Emojis in Commits
Crafting Commit Messages for Automated Tools
Well-structured messages can trigger automatic release notes or build processes, especially with CI/CD setups.
Commit Messages in Open Source Projects
Different open source communities have unique commit rules. Following them helps you blend in more smoothly. Open Source Commit Guidelines
Conclusion
Recap of Key Points
We’ve covered everything from basic structure to commit message best practices and advanced techniques for git commit messages. Solid commit messages:
- Help colleagues review and understand the history of changes
- Speed up bug tracing and new team member onboarding
- Integrate seamlessly into automation, pipelines, and versioning
Importance of Consistent Commit Messages
When commit messages follow a uniform style, the entire project benefits from easier reviews, better collaboration, and improved troubleshooting. Adopting these commit message conventions—whether you follow a strict git commit message convention or a simpler approach—can unify how changes are tracked.
Ongoing Improvement and Adaptation
- Review Practices Regularly: Check if your message guidelines fit your current workflow.
- Collect Team Feedback: Let everyone weigh in on improvements.
- Adopt New Tools or Techniques: Try out new automation services or updated standards.
- Commit to Excellence: See commit messages as a key part of quality development, not just an afterthought.
By keeping these points in mind, you transform your commit history into a robust asset for the entire team. If you spot inconsistencies, feel free to fix commit message contents, change commit message structures to match current rules, or update commit message details as your project evolves.
Appendix
Glossary of Terms
- Commit: A set of changes recorded in a Git repository at a specific point in time.
- Commit Message: Text accompanying a commit that describes the changes and their motivation.
- Conventional Commits: A simple standard for commit messages based on specific prefixes (feat, fix, docs, etc.).
- CI/CD: Continuous Integration and Continuous Deployment/Delivery, automating builds, tests, and releases.
- Semantic Versioning: A versioning method using major.minor.patch to reflect the nature of changes.
Additional Resources and References
Templates and Checklists for Git Commit Messages
Use these to keep your commit messages structured, clear, and valuable over the long haul. They’ll help you maintain high standards and streamline development in any project.