- Published on Thursday, October 31, 2024, last updated
The Core Principles of Writing a Clean Code
- Authors
- Name
- Arthur Coudouy
- @arthurcoudouy
In a world dominated by software, writing clean code is vital—not only for individual developers but for entire organizations. Clean code is not just about style; it directly affects code maintainability, scalability, and efficiency, all of which are essential in today’s dynamic tech environment.
This guide explores clean code principles that elevate both the functionality and readability of code, making it more maintainable and scalable. Whether you're writing code on your own or within a team, mastering these principles is a cornerstone of coding best practices and will benefit projects long-term. Let’s break down the key techniques to make code readable, reusable, and robust.
Table of Contents
What Is Clean Code?
At its essence, clean code is code that’s straightforward to understand, simple to modify, and efficient to maintain. This approach goes beyond mere formatting; it’s about building clean code with strong structure and scalability, ensuring that others can follow the logic without confusion.
Clean coding emphasizes readability over complexity, allowing developers to collaborate smoothly, minimize bugs, and enable quick updates. Investing in writing clean code today not only simplifies code review processes but also ensures a more future-proof, stable application.
Key Principles for Writing Clean Code:
- Simplicity - Avoid convoluted logic. Clear, simple code is easier to maintain and scale.
- Consistency - Use consistent naming and structuring. Code should be readable and predictable.
- Clarity Over Cleverness - Optimize for readability. Smart, complex solutions should not come at the cost of clarity.
- Code Review Automation - Utilize automated code review tools to enforce standards and help you understand how to review code.
Using code review automation tools allows teams to streamline the review process, making it easy to ensure clean code principles are upheld. These tools, coupled with manual code review practices, are invaluable in maintaining high standards. These code review automation tools helps maintain consistency and uphold coding standards across the entire codebase.
Example of Clean vs. Unclean Code
Consider a simple function to calculate the total price with tax:
Unclean Code:
function calc(a, b) {
return a + a * b
}
let x = calc(100, 0.2)
In this version, the code uses vague variable names (a
, b
, and x
), making it unclear what the function does and what each parameter represents.
Clean Code:
function calculateTotalPrice(basePrice, taxRate) {
return basePrice + basePrice * taxRate
}
let totalPrice = calculateTotalPrice(100, 0.2)
In this example, descriptive function and variable names help improve readability and maintainability, allowing future developers to understand calculateTotalPrice
without extra explanation.
Key Factors to Consider in Code Development
When writing code, focusing on essential principles can enhance code quality, maintainability, and collaborative effectiveness. Addressing these factors early on helps avoid technical debt and reduces the time needed for code reviews or updates. These principles will help you understand how to improve code quality.
Factor | Description |
---|---|
Readability | Code should use meaningful names and a logical structure, making it intuitive for others to follow. |
Simplicity | Streamline logic to avoid overcomplication; each line should add clear value, making maintenance easier. |
Consistency | Adhere to coding conventions to ensure a unified style that all contributors can easily understand. |
Scalability | Design code to handle future requirements, facilitating updates with minimal rework. |
Efficiency | Aim for optimized execution, reducing load times and conserving system resources for better performance. |
These foundational principles ensure that code is functional, resilient, and prepared for long-term use and collaboration. Clean coding is an investment.
Why Clean Code Matters
Even if you’re not too fond of your coworkers, you should write clean code—if only for your future self! Imagine your manager asking you to explain a tangled mess of code you wrote months ago. Clean code saves you from that awkward moment and so much more.
There are three core reasons why clean code is crucial:
Improves Code Maintainability: Writing clean code keeps the codebase organized and simple to update. This makes future modifications more manageable. Trust me, you don't want to refactor dirty code.
Facilitates Collaboration: Clear, well-organized code helps team members collaborate effectively. Managing clean code is far easier that and helps accelerating project progress.
Reduces Technical Debt: Emphasizing clean code from the start prevents the buildup of technical debt.
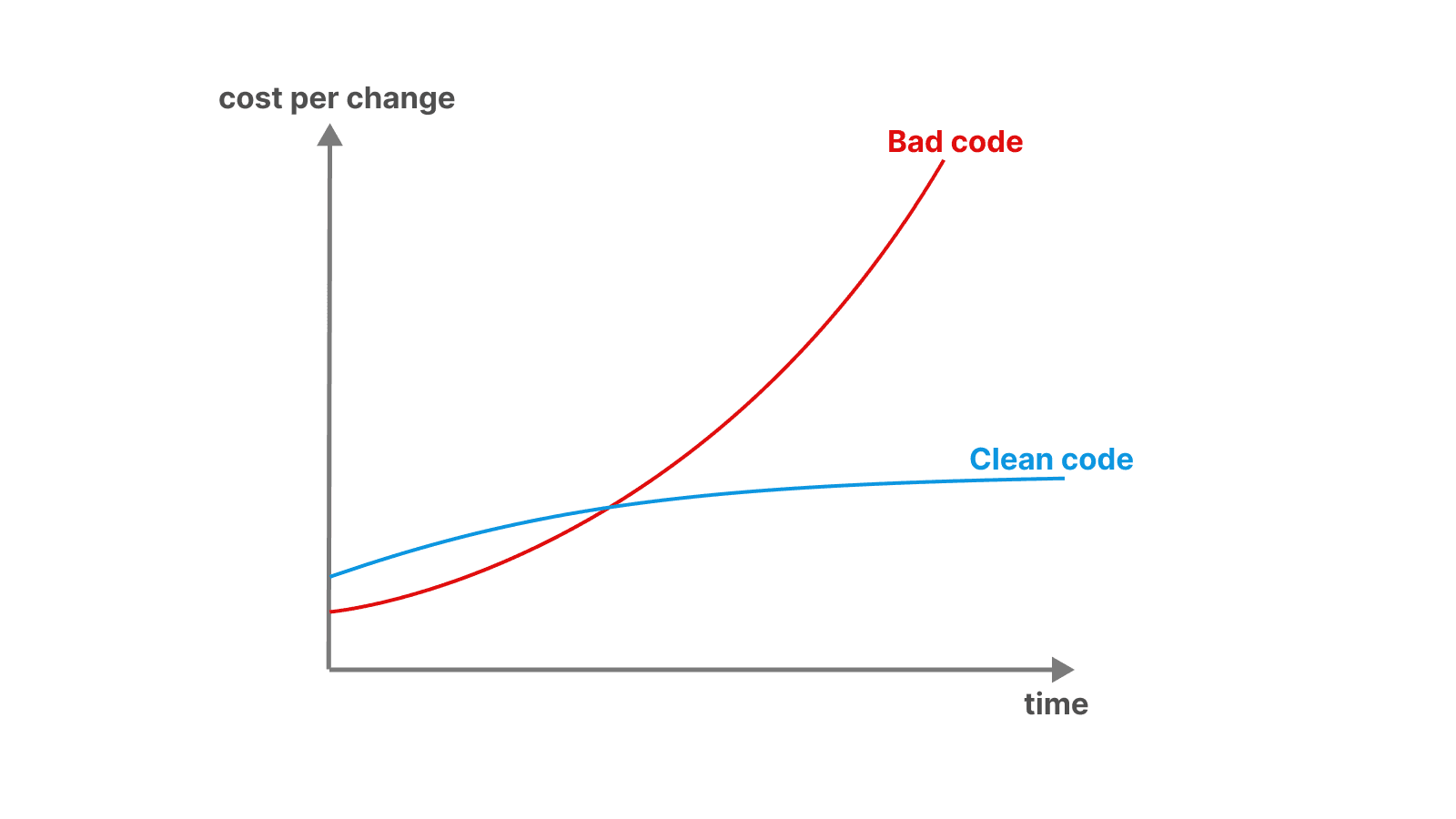
Writing clean code helps maintainability, it's a long term investment.
Core Principles of Writing Clean Code
Mastering clean code principles is essential for developers to create maintainable and scalable codebases. These guidelines go beyond mere rules—they’re actionable strategies to enhance code readability and maintainability, benefiting both you and your team. You need to understand them to learn how to improve code quality.
In this section, we’ll explore essential principles of clean coding, from effective naming conventions to reducing redundancy, all aimed at helping you write code that’s straightforward to understand, modify, and extend. If you want to go further and learn more about code security, you should also read our code review security checklist.
1. Avoid Using Hard-Coded Numbers
Hard-coding numbers directly into your code makes it difficult to understand and update values later. Instead, use constants or variables with meaningful names that describe their purpose.
Example of Hard-Coded Numbers (Not Clean Code):
function calculateTotal(price) {
return price + price * 0.2 // What does 0.2 represent?
}
In this code, 0.2
is a "magic number"—it’s unclear what it represents, which could lead to confusion if the tax rate changes.
Clean Code Example:
const TAX_RATE = 0.2
function calculateTotal(price) {
return price + price * TAX_RATE
}
By using TAX_RATE
as a constant, the code is now more understandable. If the tax rate changes, you only need to update it in one place, not everywhere else.
2. Assign Meaningful and Descriptive Names
Unclear or abbreviated names in your code make it difficult for others (and even your future self) to understand its purpose. Why not using names that clearly convey the role of the variables? You future self will thank you, and it will help your team on how to review code.
Example of Unclear Naming (Not Clean Code):
function calc(n) {
return n * 1.2 // What is 'n'? What does 1.2 represent?
}
let x = calc(100)
In this code, calc
and n
provide no context, making it difficult to understand what the function does or what value x
represents.
Clean Code Example:
function calculatePriceWithTax(basePrice) {
const TAX_MULTIPLIER = 1.2
return basePrice * TAX_MULTIPLIER
}
let totalPrice = calculatePriceWithTax(100)
Now, both the function and variables have descriptive names, making the purpose of each element clear without additional comments.
3. Keep Comments Minimal, But Make Them Meaningful
While comments can help explain code, overusing them or adding unnecessary comments can clutter your codebase. Aim to use comments only when necessary—typically to clarify complex logic or unusual decisions—and make sure they add real value.
Example of Excessive Comments (Not Clean Code):
// This function calculates the price with tax
function calculateTotal(price) {
// Multiply the price by 1.2 to include tax
return price * 1.2 // 1.2 represents the tax multiplier
}
Here, the comments are redundant because the function and variable names already make the purpose clear.
Clean Code Example:
function calculateTotal(price) {
const TAX_MULTIPLIER = 1.2
return price * TAX_MULTIPLIER
}
// Tax multiplier may change depending on region-specific rules.
Now, there’s only one meaningful comment explaining a potential reason for change, adding relevant context without repeating what the code itself expresses.
Enable your team to mergepull requests faster with Axolo
4. Keep Functions Focused
A function should handle only one clear task. When functions take on multiple roles, they become harder to read, test, and troubleshoot. Each function should have a dedicated single purpose to make your code simpler to maintain.
Example of Unfocused Function (Not Clean Code):
function processOrder(order) {
// Calculate total price with tax
const total = order.price * 1.2
// Update inventory
inventory[order.item] -= order.quantity
// Send confirmation email to customer
sendEmail(order.customerEmail, `Order for ${order.item} confirmed.`)
return total
}
This function handles multiple tasks: calculating the total, updating the inventory, and sending an email, making it harder to understand and maintain.
Clean Code Example:
function calculateTotalPrice(price) {
const TAX_MULTIPLIER = 1.2
return price * TAX_MULTIPLIER
}
function updateInventory(item, quantity) {
inventory[item] -= quantity
}
function sendConfirmationEmail(email, item) {
sendEmail(email, `Order for ${item} confirmed.`)
}
// Usage
const total = calculateTotalPrice(order.price)
updateInventory(order.item, order.quantity)
sendConfirmationEmail(order.customerEmail, order.item)
By dividing tasks into individual functions, each function has a clear, singular responsibility, resulting in more modular and manageable code.
5. Follow the DRY Principle
The DRY (Don’t Repeat Yourself) principle aims to reduce redundancy. It ensures that when you're writing code, each piece of knowledge or logic is defined only once in your codebase. This prevents duplicated code to lead to errors and make maintenance more complex.
Example of Violating DRY (Not Clean Code):
function calculateFinalPriceWithTax(price) {
return price * 1.2
}
function calculateDiscountedPriceWithTax(price, discount) {
const discountedPrice = price - price * discount
return discountedPrice * 1.2 // The tax multiplier is repeated
}
In this example, the tax multiplier 1.2
is duplicated across functions, which increases the risk of inconsistencies if the tax rate changes.
Clean Code Example:
const TAX_MULTIPLIER = 1.2
function calculateFinalPriceWithTax(price) {
return price * TAX_MULTIPLIER
}
function calculateDiscountedPriceWithTax(price, discount) {
const discountedPrice = price - price * discount
return discountedPrice * TAX_MULTIPLIER
}
By defining a single TAX_MULTIPLIER
constant, redundancy is minimized. If the tax rate changes, it only needs to be updated in one location.
6. Adhere to Established Code-Writing Standards
If you want to make it easier for your team to collaborate and for new developers to undersand your code, you should follow established coding standards. They ensure consistency throughout the codebase. These standards usually cover naming conventions, formatting practices, and structural guidelines.
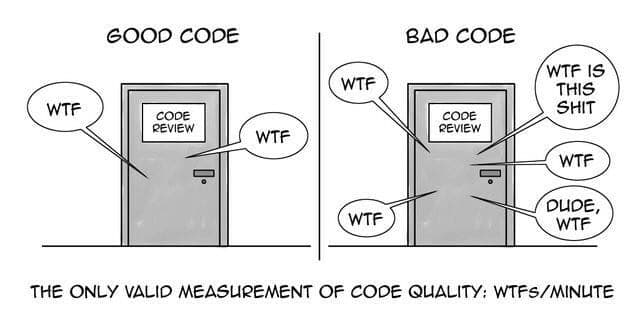
Prevent a high wtf/minute ratio during code reviews with clean code.
Example of Inconsistent Coding Standards (Not Clean Code):
function calculate_total(price) {
return price * 1.2
}
function UpdateInventory(item, quantity) {
inventory[item] -= quantity
}
Do you see why in this example, inconsistent naming conventions and formatting (like varying use of camelCase and snake_case, and irregular spacing) make the code harder to read? Let's try to improve it.
Clean Code Example:
function calculateTotal(price) {
return price * 1.2
}
function updateInventory(item, quantity) {
inventory[item] -= quantity
}
In this example, the code consistently applies camelCase for function names and maintains uniform spacing and indentation. Following these standards enhances readability and helps all team members easily understand the code.
Axolo is a Slack app to help techteams review pull request seamlessly
7. Encapsulate Nested Conditionals into Functions
Nested conditionals make code difficult to read and comprehend. You need to simplify the main code flow by encapsulating complex conditionals into well-named functions.
Example of Nested Conditionals (Not Clean Code):
function processOrder(order) {
if (order.status === "paid") {
if (order.items.length > 0) {
if (order.shippingAddress) {
shipOrder(order)
}
}
}
}
In this code, the nested conditionals obscure the logic. It's hard to quickly understand under what conditions an order is shipped.
Clean Code Example:
function isReadyToShip(order) {
return order.status === "paid" && order.items.length > 0 && order.shippingAddress
}
function processOrder(order) {
if (isReadyToShip(order)) {
shipOrder(order)
}
}
By moving the conditional logic into a descriptive function, isReadyToShip
, we’ve made the processOrder
function more readable. This approach also makes the code easier to test and maintain, as the complex logic is isolated in one place.
8. Continuously Refactor
Even if your colleagues do not like refactoring, it keeps the codebase clean and efficient. But do you know what refactoring is? It is the process to reorganize existing code to enhance its readability, maintainability, or performance without altering its purpose.
Example of Code Needing Refactoring (Not Clean Code):
function createUser(firstName, lastName, age, email) {
const user = {}
user.firstName = firstName
user.lastName = lastName
user.age = age
user.email = email
user.fullName = firstName + " " + lastName
return user
}
In this example, the function has repetitive steps for setting each property, and the fullName
creation is mixed in with the other code, which could be improved.
Clean Code Example (After Refactoring):
function createUser(firstName, lastName, age, email) {
const user = { firstName, lastName, age, email, fullName: getFullName(firstName, lastName) }
return user
}
function getFullName(firstName, lastName) {
return `${firstName} ${lastName}`
}
Extracting getFullName
into a separate function simplifies createUser
, making it cleaner and more focused. Regularly refactoring code in this way reduces complexity, ensuring it stays easy to understand and extend.
9. Utilize Version Control
Version control is crucial for tracking changes, and also preserving a history of your codebase. It allows you to experiment with new features, revert to earlier versions when necessary, and review changes efficiently.
Example of Not Using Version Control (Not Clean Code):
project_v1/
project_v2/
project_final_v3/
project_final_FINAL_v4/
This manual approach to version tracking is unreliable, confusing, and error-prone. It’s hard to identify which version is the latest and manage changes across multiple developers.
Clean Code Example (Using Version Control):
# Initialize a git repository
git init
# Stage and commit changes
git add .
git commit -m "Initial commit"
# Create a branch for a new feature
git checkout -b feature/add-user-authentication
# Merge feature branch after testing
git checkout main
git merge feature/add-user-authentication
With version control (e.g., Git), changes are tracked automatically, and branching allows you to work on features independently. Using descriptive commit messages and branches makes collaboration and code history management easy and efficient.
Best Practices for Writing Clean Code
Applying clean coding principles requires consistent effort and attention to detail. These best practices ensure that your code remains readable, maintainable, and efficient as it grows. Here are essential habits that help developers produce cleaner code.
Use Consistent Code Formatting
Consistency in formatting isn't just about aesthetics—it's essential for efficient collaboration. Use tools like Prettier or ESLint to automate style enforcement while writing code, making your codebase cohesive and easy for any team member to follow.
Write Small, Reusable Components
Break down complex functionality into single-purpose functions or components. By focusing each function on a specific task, you enhance reusability and make testing far easier, allowing you to adapt parts of your code effortlessly across the project.
Write Tests and Use TDD (Test-Driven Development)
Testing code as you write it saves time in the long run. TDD encourages you to start with tests, ensuring every feature works as expected. Automated tests guard against errors, enabling refactoring and new development without worry of breaking existing functionality.
Prioritize Readability Over Cleverness
Readable code makes life easier for everyone. Opt for clear, simple solutions rather than complex "clever" tricks. Code that’s easy to read can be understood without extra explanations, saving time during reviews and minimizing confusion in collaborative environments.
Document Wisely
While comments should be kept minimal, certain parts of the code—such as complex logic or APIs—benefit from clear documentation. Avoid over-commenting and instead document only where it adds real value. This could include explaining specific business logic, describing function purposes, or adding information on dependencies.
Review Code Regularly
Frequent peer code reviews are essential for maintaining code quality and ensuring clean code principles. Because it helps sharing knowledge, and identifying issues early. Code reviews foster a collaborative culture that promotes continuous improvement.
By adopting a good code review practice, developers can produce code that is easier to understand, and more reliable.
Tools and Resources to Help Write Clean Code
Using the right GitHub or GitLab code review tools and resources can make it easier to implement and maintain clean code standards and follow coding best practices. Automation, formatting tools, and version control all contribute to a more efficient workflow, ensuring code quality without excessive manual effort. Leveraging code review tools effectively can help you ensure every line of code adheres to established standards.
Automate Code Reviews with Axolo
Do you receive a lot of GitHub request review from your team?
Axolo integrates seamlessly with GitHub and Slack to automate code reviews and improve team collaboration as a code review automation. By creating a dedicated Slack channel for each pull request, Axolo enables team members to discuss changes, suggest improvements, and quickly resolve issues, all in real time. With Axolo, you can streamline the review process, ensuring that every pull request aligns with clean code standards. Axolo also helps ensure velocity on how to review code with features such as daily pull request reminders.
Key Benefits of Axolo:
- Improves Communication: Axolo’s Slack integration allows for fast feedback and discussion, ensuring everyone involved is up-to-date and can contribute effectively.
- Enhances Code Quality: Automated code review reminders help maintain consistent review standards, preventing issues before they reach production.
- Saves Time: Axolo reduces the time spent on managing pull requests by automatically notifying the right team members, minimizing unnecessary distractions.
Clean code is an ongoing effort, especially in collaborative projects. Axolo helps teams maintain code quality by integrating code review automation directly into their workflows. With Axolo, teams can enforce clean code practices effortlessly, communicate effectively on pull requests, and keep the codebase maintainable and robust. By automating reviews, code review tools like Axolo streamline processes and ensure high-quality code across projects.
If you're interested, you should learn more with the top GitHub Slack integrations!
Axolo User Experiences
2480+ developers online
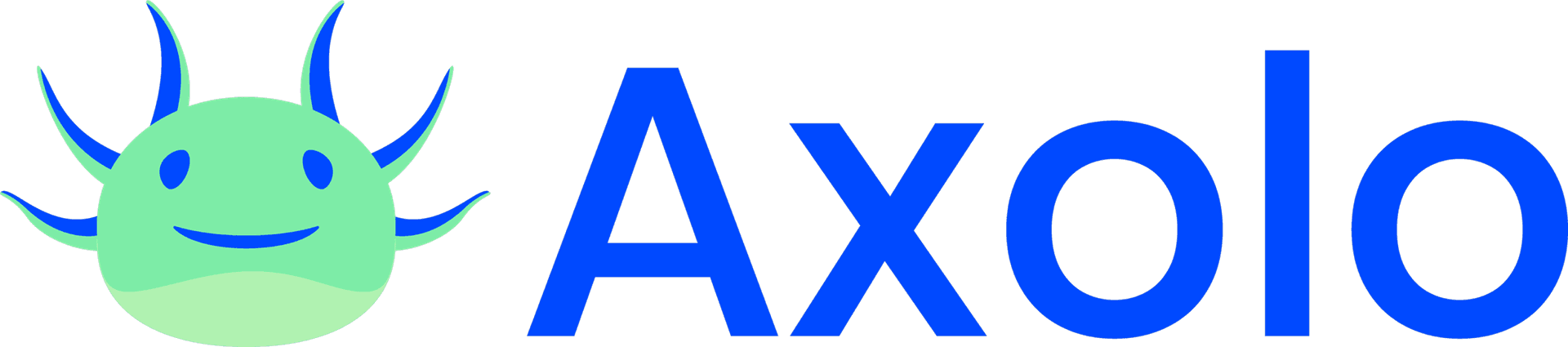
Code quality has improved, engineers have a better understanding of what their colleagues are working on, and code is getting shipped much faster. Time from pull request open to review to merge is WAY faster, and engagement with reviews is much higher for our team. Axolo's team is also unbelievably fast in resolving issues when we run into them. Pleasure to work with them.
Axolo is an incredible tool for connecting PRs with our everyday engineering discussion on Slack. It integrates seamlessly and makes it so as a manager, I never lose track of my engineers' work. For my engineers, it allows them to collaborate without losing a thread in different contexts. Overall it keeps us moving swiftly throughout the sprint and continuously reviewing and merging code!
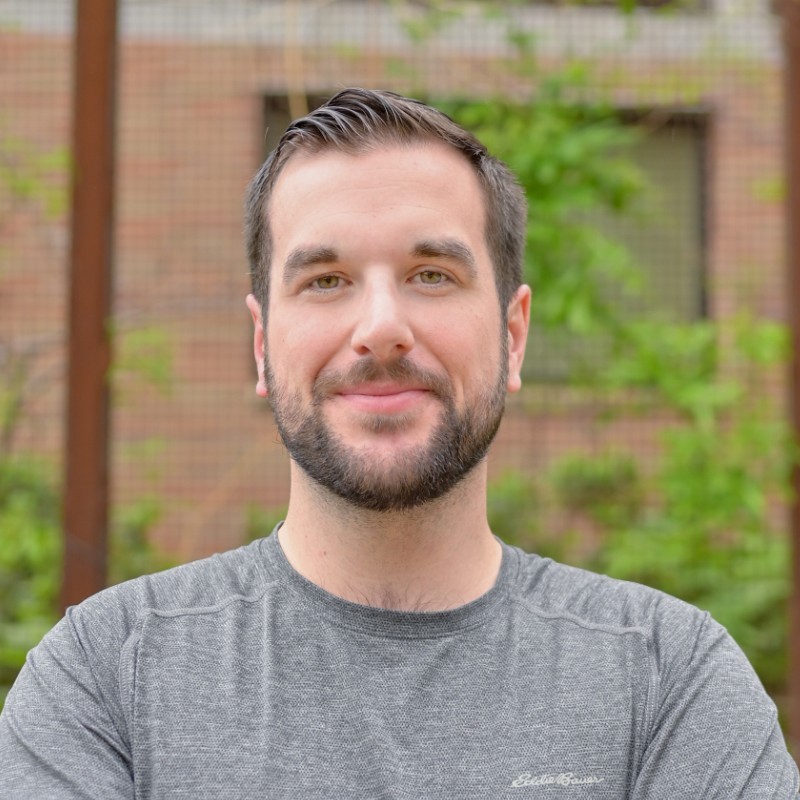
Axolo has made it easier to hold developers and QA engineers accountable for reviewing and merging pull requests on a timely basis. Our average PR time-to-merge was 2.40 days before Axolo, this has been reduced to 1.51 days after only using it for 2 weeks.