Axolo User Provisioning and Deprovisioning API
This documentation provides the API details for automating user provisioning and deprovisioning for enterprise customers.
3 Steps to Enable API Access
- Contact Support: Contact Axolo support to enable API access for your account.
- Create an API Key: Once API access is enabled, generate an API key from the Axolo app dashboard in the Settings/API Key menu.
- Follow the Documentation: Use the following endpoints to make onboarding and offboarding API calls.
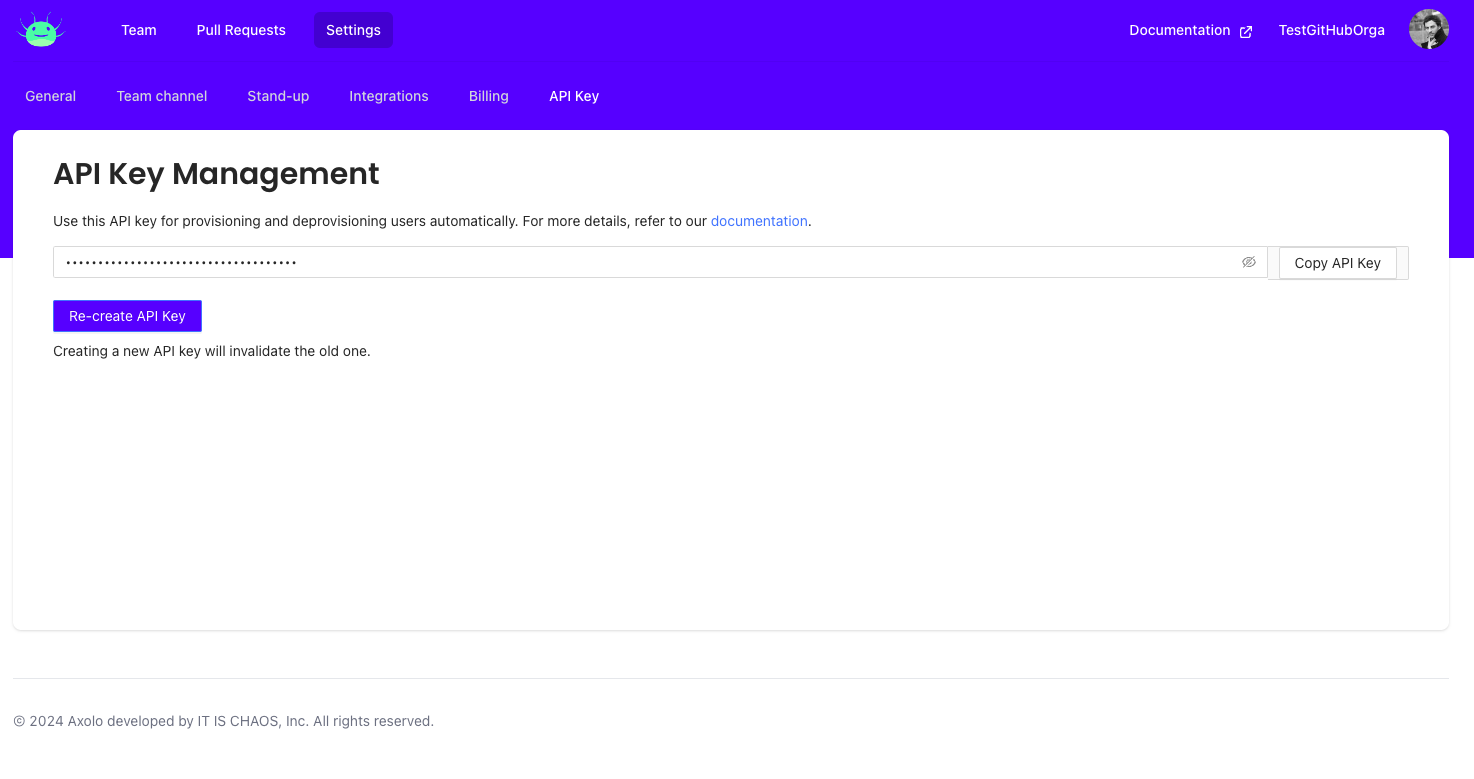
API Endpoints
Onboard User
Endpoint: POST https://api.axolo.co/v1/engineer/onboard
Headers:
Content-Type: application/json
x-api-key: YOUR_API_KEY
Request Body:
{
"organization": "org_name", // name of your GitHub organization, this is case sensitive
"githubLogin": "user123", // name of the github user you want to onboard
"slackId": "U12345678" // Slack ID of the corresponding user
}
Example cURL Request:
curl -X POST https://api.axolo.co/v1/engineer/onboard \
-H "Content-Type: application/json" \
-H "x-api-key: YOUR_API_KEY" \
-d '{
"organization": "org_name",
"githubLogin": "user123",
"slackId": "U12345678"
}'
Example JavaScript Request:
const axios = require('axios')
const data = {
organization: 'org_name',
githubLogin: 'user123',
slackId: 'U12345678',
}
axios
.post('https://api.axolo.co/v1/engineer/onboard', data, {
headers: {
'Content-Type': 'application/json',
'x-api-key': 'YOUR_API_KEY',
},
})
.then((response) => {
console.log('User provisioned successfully:', response.data)
})
.catch((error) => {
console.error(
'Error provisioning user:',
error.response ? error.response.data : error.message
)
})
Offboard User
Endpoint: POST https://api.axolo.co/v1/engineer/offboard
Headers:
Content-Type: application/json
x-api-key: YOUR_API_KEY
Request Body:
{
"organization": "org_name",
"githubLogin": "user123"
}
Example cURL Request:
curl -X POST https://api.axolo.co/v1/engineer/offboard \
-H "Content-Type: application/json" \
-H "x-api-key: YOUR_API_KEY" \
-d '{
"organization": "org_name",
"githubLogin": "user123"
}'
Example JavaScript Request:
const axios = require('axios')
const data = {
organization: 'org_name',
githubLogin: 'user123',
}
axios
.post('https://api.axolo.co/v1/engineer/offboard', data, {
headers: {
'Content-Type': 'application/json',
'x-api-key': 'YOUR_API_KEY',
},
})
.then((response) => {
console.log('User deprovisioned successfully:', response.data)
})
.catch((error) => {
console.error(
'Error deprovisioning user:',
error.response ? error.response.data : error.message
)
})